Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial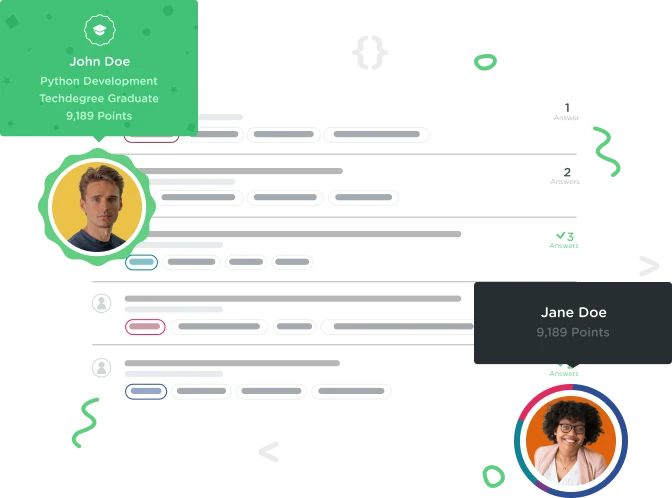
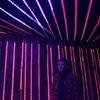
Muhammad Bobojonov
6,325 PointsWhy React class component is not receiving passed props?
I am working on a personal project. When I pass props from functional component to class component, I am getting an empty array. I have tested it with other test variables (such as plain string), but still class component doesnt receive it.
Please Help.
Here is functional component:
import React from "react" import ProjectList from './ProjectList' import { useStaticQuery, graphql } from "gatsby"
const getProjects = graphql
query {
projects: allContentfulProject {
edges {
node {
name
description {
description
}
short
location {
lon
lat
}
province
mainPic {
fluid {
...GatsbyContentfulFluid_tracedSVG
}
}
slug
}
}
}
}
const Projects = () => { const {projects} = useStaticQuery(getProjects) const test = "hello" console.log(projects);
return <ProjectList test={test} /> }
export default Projects
And HERE IS CLASS Component:
import React, { Component } from "react" import styles from "../../css/items.module.css" import Project from "../Projects/Project"
export default class ProjectList extends Component { state={ projects:[], sorted:[] }
componentDidMount(){ this.setState({ projects: this.props.projects.edges, sorted: this.props.projects.edges }) } render() { return ( <section className={styles.tours}> <div className={styles.center}> { this.state.sorted.map(({node})=>{ return <Project key={node.slug} project={node}/> }) } </div> </section> ) } }
2 Answers

Torben Korb
Front End Web Development Techdegree Graduate 91,432 PointsHi Muhammad, to make your code better readable in here you can use triple backticks (```) around your code and you get syntax highlighting. Check the "Markdown Cheatsheet" link below the answer textbox. When your code's more readable it's more easy for others to follow your code.
By just quick glancing at your code it seems to me that you only pass the "test" prop to your class component (ProjectList) which only holds a string with the value "hello". To access this prop you would need to write this.props.test ... anyway it doesn't give you the desired behavior. To assign the projects correctly you would assign it something like this for example:
// In functional component
<ProjectList projects=projects />
And in your class component you would access this prop like this:
// inside the class component
this.setState({
projects: this.props.projects,
...
});
Whatever you reach down the line can be accessed with the property key you assigned it before. Hope this is understandable and helps you! Happy coding!
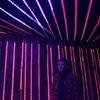
Muhammad Bobojonov
6,325 PointsGot it, thank you for your help :)