Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial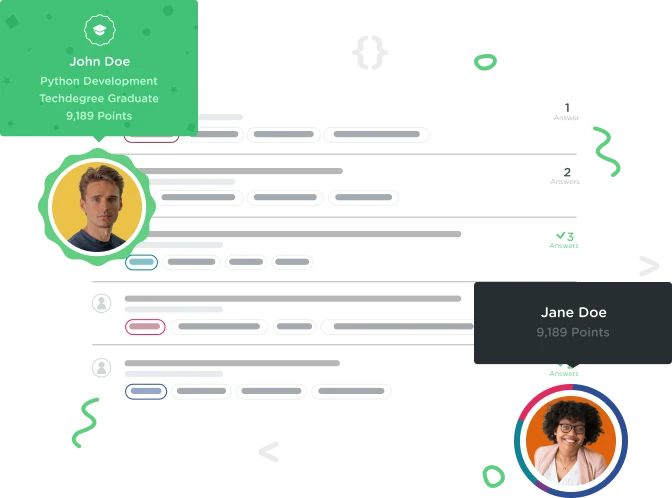

Vitaly Khe
7,160 PointsWhy refactoring getQuotes to async/await doesn't work?
const getQuotes = async () => fileSystem.readFile('data.json', 'utf8', (err, data) => {
if (err) {
return err;
}
else {
const quotes = JSON.parse(data);
return quotes;
}
});
(async () => {
const q = await getQuotes();
console.log( q);
})();
Console:
Promise { undefined }
2 Answers
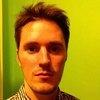
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsThe readFile
method doesn't return anything. If you have a function that takes a callback, and you want it to return a promise, one strategy is to wrap it in a Promise constructor, which takes a function with two arguments, resolve
and reject
. You can call resolve()
on the successful response and reject()
on the error.
const getQuotes = () => {
return new Promise((resolve, reject) => {
fileSystem.readFile('data.json', 'utf8', (err, data) => {
if (err) {
return reject(err);
} else {
const quotes = JSON.parse(data);
return resolve(quotes);
}
})
})
}
You can also use a neat utility function called promisify

Vitaly Khe
7,160 PointsGot it, thanks
karan Badhwar
Web Development Techdegree Graduate 18,135 Pointskaran Badhwar
Web Development Techdegree Graduate 18,135 PointsHey Brendan Whiting, sorry to post a question to your answer so late. But she said
transform the getUsers function into a promise if it wasn't one
, if we add async in front of the function, then again why do we need to return a Promise, if it's gonna do that for us?