Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial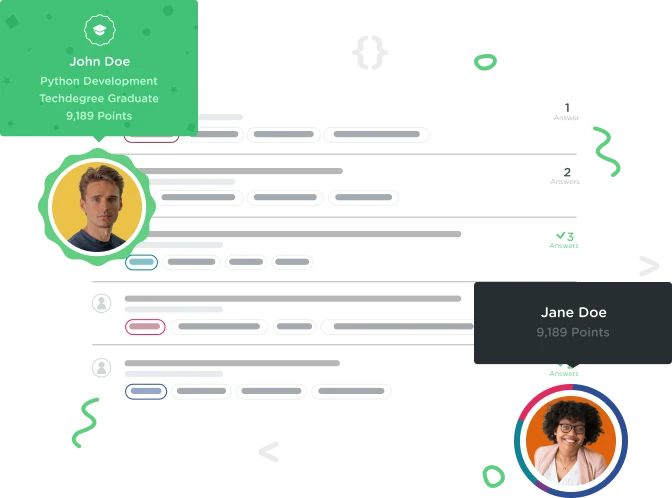

chailatte
941 PointsWhy return a string?
def just_right(string):
if len(string) < 5:
return "Your string is too short"
elif len(string) > 5:
return "Your string is too long"
else:
return True
From my understanding, the return function is used to return a value to do some additional calculations. I can see how this applies for number values but what calculation (other than string concatenation) would you do with a string as in this CC?
Bonus: How do I share my code (with those gray boxes) especially for a CC when I post here on Community? For this one I had to re-enter code into Workspaces, take a snapshot, and then copy the link to it.
1 Answer

andren
28,558 PointsValues are not returned just to do calculations, that's just one example of what you can do. The return
statement is essentially just a reverse example of passing an argument into a function. The function is giving a value back to the code that called it and once returned the value can be used for anything that value normally could be.
Let's say you had a function that was designed to present the user with a list of options and then asked the user to choose one of them. That function would not be very useful if i did not tell the code that called it what the user actually ended up choosing, which it can easily do by returning the choice.
Also consider built-in methods in Python like upper
(uppercase a string) and lower
(lowercase a string) those are methods that take a string and then returns a string that has had specific formatting applied to it. They would not serve much use if they did not ultimately return the string they created from the string you called them on.
You can return literally any value in Python, not just strings and numbers. And as you get farther into the courses you'll start to get a better understand of why that is a useful. There are many functions where the function serves no real purpose if it does not actually return the value it worked on after it has finished.
As for your question on code formatting, there is a link below the box where you write your comment labeled "Markdown Cheatsheet" which when clicked opens up a window with various formatting tips. For code formatting you simply need to write three backticks ``` then the name of the language you are embedding followed by a new line. Then paste in your code, then on a new line below your code enter three more backticks.

chailatte
941 PointsThank you for the explanation. I think I'm starting to get the hang of it.
However, in this particular CC I wonder why you would want to return "Your string is too long/short." It would appear that a string like that would be intended for the user to see. Unless this code is part of a longer code where other things would be done based on those strings.

andren
28,558 PointsWell in that particular case it's more just that the challenge checker deals better with returned values, as it makes it very easy to check that your function produces the right output. It also serves to train people on how to return a value, which is needed since there tends to be a decent number of students who confuse return
and print
at this point in the course.
The challenges are often contrived code examples made to test your knowledge of a specific thing you just learned and are expected to know, rather than code that servers a useful purpose.
Edit:
But as you stated this could also theoretically be code that takes those strings and still end up presenting them to the user, but in more complex way. Say for example that you were writing an application that had both a console UI and a graphical UI. In that case you might want to separate all of the logic code of your application from the code that ultimately displayed anything. So this just_right
function could be called from a function which then passed the string along either to a function designed for console output or for GUI output.

chailatte
941 PointsGotcha. Thanks again for responding!
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsI think you understanding the return statement is limited as I would be asking why can't I return a string, just as there are unlimited use cases for functions, there are also unlimited use cases for returning strings.
For code sharing and other "Markdown" in your post, checkout the "Markdown Cheatsheet" link under the textarea.
When I want to insert code, like the example below, Use 3 lines. Line 1 - Three backtics (
- key to the left of the 1 and above the tab on a standard us keyboard) and language (like python) Line 2 - blank Line 3 - Three closing backtics (
) Then I insert my code into the blank line 2Example: