Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial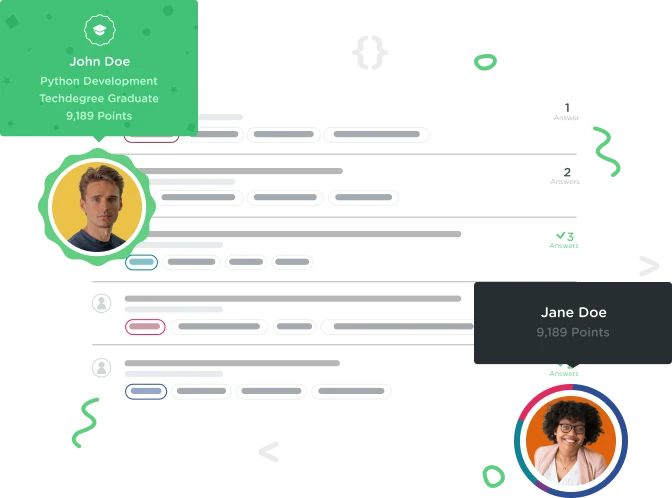

James Love
1,279 PointsWhy return Bool in exercise?
I don't see why you wouldn't get the function to complete the task fully. Is this code incorrect?
func isDivisible(#number1: Int, #number2: Int) -> String {
if number1 % number2 == 0 {
return "Divisible"
} else {
return "Not Divisible"
}
}

Matthias Schlemm
Courses Plus Student 47 PointsWell it's not really verbose, printing the result is only for testing, you don't really want a String, you want to know whether two numbers are divisible and that's a true or false question. It shouldn't be an optional either since number1 and number2 aren't optionals either. You also need have to check whether number 2 is zero or not, or you'll divide by zero

James Love
1,279 PointsThanks Matthias,
I was being dense - it's been a long day!
1 Answer

Geoff Parsons
11,679 PointsAs Matthias Schlemm pointed out it's much easier to build a conditional around a function that returns a boolean than it is to evaluate a string return.
if( isDivisible(number1: 1, number2: 0) ) {
// do something
}
if( isDivisible(number1: 1, number2: 0) == "divisible" ) {
// do something
}
On top of producing easier to read code it's also more efficient. Booleans take up very little space in memory and comparing a boolean is a more efficient operation than comparing two strings. In general if your function asks a true/false question it should return a boolean.

James Love
1,279 PointsGreat! Thanks Geoff. Completely makes sense that it would be easier to use later and that it saves power to compute in more complex situations. Can't believe I didn't notice that earlier really!
James Love
1,279 PointsJames Love
1,279 PointsThanks Matthias but I am not sure I understand you. Comparing my example of code with one I have just amended to reflect the "answer" given by Amit:
I find that this "correct" version is verbose and I can't see a difference in functionality. Is there a reason my original code couldn't be used in another scenario?