Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial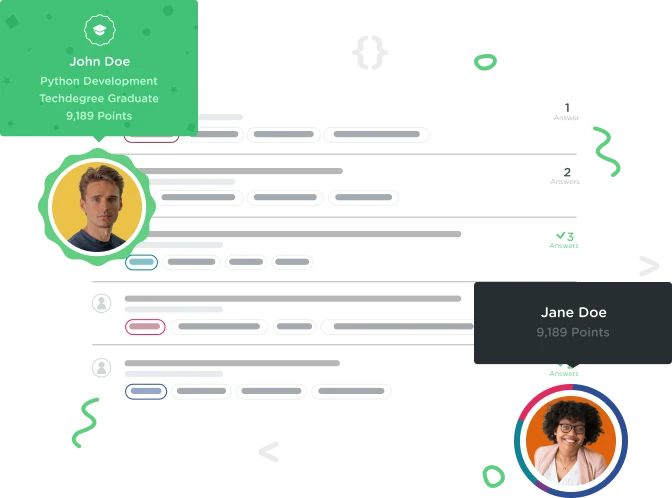
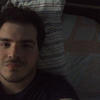
henriquegiuliani
12,296 PointsWhy should i choose between structs and classes?
I know that classes accept heritance, but which more points should i consider to decide between struct or class for a data structure? Is there any good practice/recommendation for that?
1 Answer
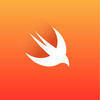
Steven Deutsch
21,046 PointsHey Henrique Giuliani,
I recommend you read the section in the Swift Programming Language Guide on Choosing Between Classes and Structures.
Some cases where you would use a struct is where:
- You are modeling a relatively simple data type. ex) a Point type, composed of an X and Y property
- Your type does not need inheritance. (Although you will learn later you can use protocols to gain inheritance like capabilities)
- You want the objects of this type to be passed by value rather than reference
It's important that you understand the difference between value types and reference types. In Swift, structs are value types and classes are reference types. When a value type is assigned to a variable or constant, its value is copied over on assignment. For example:
var number1 = 10
var number2 = number1
The Int type in Swift is a value type (implemented using a Struct). In this code, the value from number1 is copied and assigned as the value to number two. Both number1 and number2 exist as separate values in memory. Therefore, if we update the value of number1, number2 will not be changed.
var number1 = 10
var number2 = number1 // number2 is 10
number1 = 20 // number1 is now 20
print(number2) // number2 is still 10
Classes on the other hand, are reference types. This means when you assign one class to another variable or constant, what is assigned is not the copied value, but rather a reference to the original value in memory. For example:
class Person {
var name: String = "Henrique"
}
let person = Person()
print(person.name) // will print "Henrique"
let anotherPerson = person
person.name = "John"
print(anotherPerson.name) // will print "John"
Here you can see that when we assigned the instance of person to a constant called anotherPerson, the Person value was not copied when it was assigned. Instead, we created a reference to the original person object. You can see this is the case because when we change the name of the original person, the name of anotherPerson was changed as well. This is because they are not separate objects, but rather different references to the same object in memory. You can think of it like one object having two different names.
Hope this helps. Good Luck!