Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial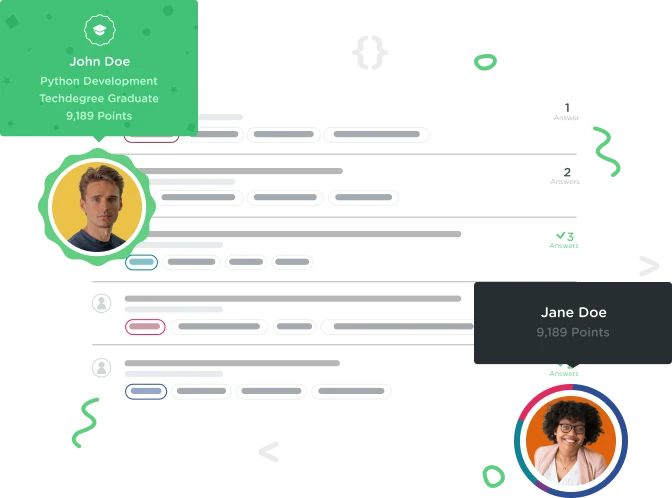

Active Man
300 Pointswhy should i throw an Exception in PHP?
I new to oop, i will like to know is it important to throw an exception, what is the actual use?
5 Answers
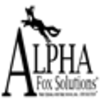
Shawn Gregory
Courses Plus Student 40,672 PointsHello,
Depending on what you are doing you may or may not need to use exceptions. When you are validating something it's always good that you use exceptions. Exceptions are there so when an exception is meet, it throws it to the catch block and prevents further code from working. This can be good when you are validating user input and at the end of the user input (say for example you are registering a user) you are saving the data and sending them to a success page. Well, does it make sense to add values to a database and send them to a success page if some of the input isn't correct? Below is an example widely used in the real world that best illustrates why you should use an exception:
if($_POST) {
try {
if(UserExists($_POST['user']) { // UserExist() function checks to see if a user with the same username exists
throw Exception('The username provided is already being used. Please try again.');
}
if($_POST['pass1'] != $_POST['pass2']) {
throw Exception('The two passwords provided do not match. Please try again.');
}
if(EmailValid($_POST['email']) { // EmailValid() function checks to see if a email is valid
throw Exception('The email provided is invalid. Please try again.');
}
SaveInfo($_POST); // SaveInfo() function saves the user input into the database
header("Location: success.php");
}
catch(Exception $e) {
$err = $e;
}
}
// Display form to user (including any errors that may have occurred)
In the above example you can see how the use of exceptions can be beneficial. You may or may not need to use exceptions in your code, but knowing how to use them and where you can use them can assist you in not having to type as much code as needed. Please look into php.net's exceptions to find out more. Hope this helps!
Cheers!

Active Man
300 PointsShawn Gregory Thanks for your great explanation. but i have never used exception before, checkout the way i use to do validation and tell me if something is wrong about it.
if($_POST) {
$error=array();
if(UserExists($_POST['user']){ // UserExist() function checks to see if a user with the same username exists
$error[]="The username provided is already being used. Please try again.";
}
if($_POST['pass1'] != $_POST['pass2']) {
$error[]="The two passwords provided do not match. Please try again.";
}
if(EmailValid($_POST['email']){ // EmailValid() function checks to see if a email is valid
$error[]="The email provided is invalid. Please try again.";
}
if(count($error)){
///// we have errors
foreach($error as $var){
echo $var . "<br />";
}
}else{
/// we do not have errors
}
}
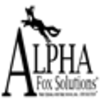
Shawn Gregory
Courses Plus Student 40,672 PointsActive Man,
Your way is fine as well. Exceptions are good when you get an error and you must act on that one error and nothing else. If you want to, however, accumulate all of your errors and then display them in a list when you redisplay the form to a user, your way will work fine (I've used your way just as many times if not more than using exceptions). Exceptions are there for you to use whether you use them or not. It's a way to handle that one error that you need to check and handle less it ends up breaking everything else on the page. Think of using exceptions to check for errors that, if the error occurs the rest of the script doesn't work. You are not required to use exceptions, but just knowing it's there and how to use it; you will gain a better programming style knowing that you can use something that will assist you in a specific circumstance. Like I said before, exceptions aren't required but knowing that they are there will help you down the road. Look here: http://php.net/exceptions for more information regarding PHP exceptions.
Cheers!

Active Man
300 PointsShawn Gregory Thanks for your reply, i think i understand now.

Casey Ydenberg
15,622 PointsThink of it this way: you want each function and class you define to be as independent as possible. This promotes code reuse and makes it easier to write programs: you don't need to have the entire codebase in your mind in order to work with it. Exceptions are an essential part of this architecture: they deal with the cases where the parameters received just do not make sense. That way, if the function is called by from multiple other places by higher-level functions, they can each handle it in whatever way makes the most sense for them.
To take a ridiculously simple example, suppose you have a function to calculate mortgage interest rates over a specified number of years. You should consider the possibility that the "number of years" parameter might ever be 0, resulting in a division error. This is especially true if this might be user input, but even if not, it is possible you or another programmer might make a mistake later. Better to check the value, and return an exception if 0 - and cease execution of the function which will cause a fatal error and an ugly error message that the user will see. The exception will make it very clear to you where things went wrong, and you can decide later how the higher level functions should gracefully pass the information onto the user.