Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial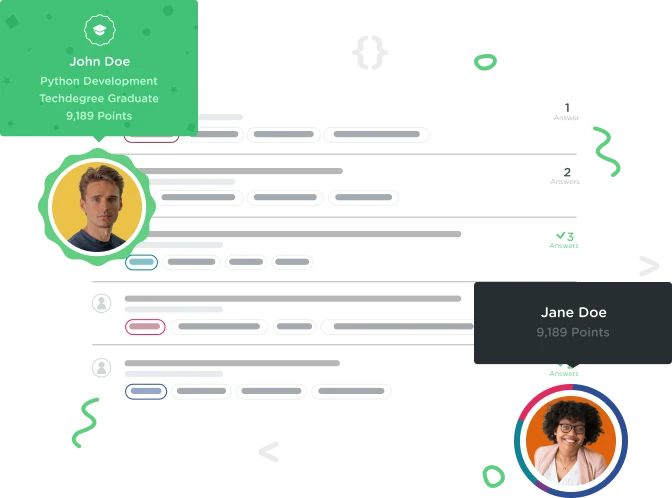

paulscanlon
Courses Plus Student 26,735 PointsWhy start on 0?
Hi. I believe starting on 0 in a list is fairly common in programming, but why? Seems like it would be something to really easily slip up on, even for an experienced programmer.
Thanks
Paul
1 Answer

Jon Mirow
9,864 PointsHI paul,
You're absolutely right, most programming languages are what's called "zero-indexed", meaning they start counting from 0.
To understand the reason why, consider the following array filled with empty arrays:
var arr = [ [], [] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ];
If you haven't seen nested arrays yet, just know you can put anything in an array - even other arrays! Here I'm just using them to show empty slots.
Suppose I want to put the value 3 in the 4th "box":
var arr = [ [], [] ,[] ,[3] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ,[] ];
So far so good, but now i want to use it for something, so I ask the computer to find for me the array holding my 3. How does the computer find it?
This array is stored in memory, and the variable name "arr" points to where it starts, so the computer first goes to the start of the array, and doesn't find anything there, so then it goes to the next element, and the next, then one more element and finds it. It had to take 3 steps forwards from the start to get to the 4th element in the array.
And it's not just trying to find something in an array, it's any time we want to get a value out or put one in, the computer has to go to the start, and then move a certain number of steps forward to find the data we're looking for. As the starting point will always be the first element of the array, the computer counts from zero, not one like how we'd count.
This all goes back to the earliest programming languages when things were very simple and you had to know exactly which address in memory held the value you wanted, and in the case of arrays, how many (and how large) steps you had to make forwards to get to where you needed.
Nowadays higher level languages like javascript could hide this from us - it'd be very trivial to make it more "human friendly", but two things - firstly that you actually get used to zero-indexed very fast, so you expect other languages to use it; and secondly it's more accurate to how the computer is running.
And yes, you're definitely right about even experienced programmers making mistakes! This is often called an "off by one" error - not accounting for the zero index and getting the wrong value, or worse, getting the next value stored in memory after the array ends. Many security exploits over the years have been the result of off by one errors! Sadly computers will always do what we tell them to, not what we meant for them to do.
I hope it helps :)
paulscanlon
Courses Plus Student 26,735 Pointspaulscanlon
Courses Plus Student 26,735 PointsThanks for your in depth reply. It really explained it