Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial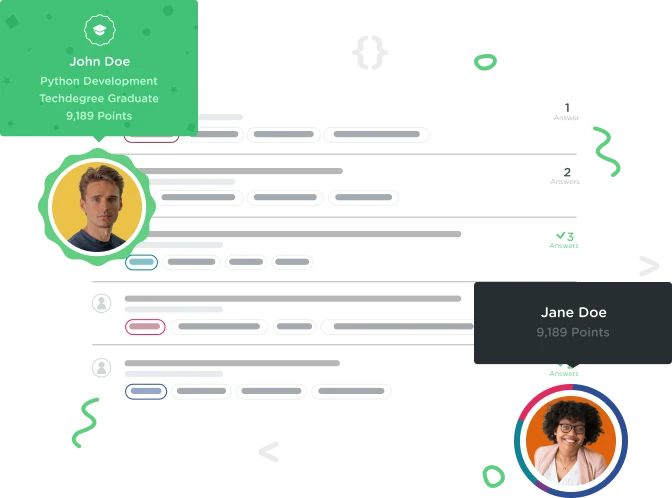

tae shik hwang
11,827 PointsWhy the error is occurred??
const mongoose = require('mongoose'); mongoose.connect('mongodb://localhost:27017/sandbox'); const db = mongoose.connection;
db.on('error', err => console.log('Connection error: ', err)); db.once('open', () => { console.log('db connection successful'); // All database communication here
const Schema = mongoose.Schema;
const AnimalSchema = new Schema({
type: {type: String, default: "goldFish"},
color: {type: String, default: "golden"},
size: String,
mass: {type: Number, default: 0.006},
name: {type: String, default: "Simon"},
});
AnimalSchema.pre('save', next => {
if(this.mass >= 100) {
this.size = 'big';
} else if(this.mass >= 5 && this.mass < 100) {
this.size = 'mdeium';
} else {
this.size = 'small';
}
next();
});
let Animal = mongoose.model('Animal', AnimalSchema);
let elephant = new Animal({
type: "elephant",
color: "gray",
mass: 6000,
name: "David"
});
let animal = new Animal({}); // glodfish
let whale = new Animal({
type: "whale",
mass: 190500,
name: "Angela"
});
const animalData = [
{
type: "mouse",
color: "gray",
mass: 0.035,
name: "Micky"
},
{
type: "nutria",
color: "brown",
mass: 0.12,
name: "Johny"
},
{
type: "wolf",
color: "yellow",
mass: 35,
name: "Ghill"
},
elephant,
animal,
whale
];
Animal.remove({}, (err) => {
if(err) console.error(err);
Animal.create(animalData, (err, animals) => {
if(err) console.error(err);
Animal.find({}, (err, animals) => {
animals.forEach(animal => {
console.log(animal.name + " the " + animal.color +
" " + animal.type + " is a " + animal.size + "-sized animal.");
}); // animals.forEach
}); // Animal.find
db.close(() => console.log('db connection closed'));
}); //Animal.create
}); //Animal.remove
}); // db.on
TypeError: Cannot read property 'forEach' of undefined at Animal.find (/Users/ian/Desktop/icloud/pgm/treehouse/javascript/qa-rest-api/mongoose_sandbox.js:84:24) at /Users/ian/Desktop/icloud/pgm/treehouse/javascript/qa-rest-api/node_modules/mongoose/lib/model.js:3433:16 at /Users/ian/Desktop/icloud/pgm/treehouse/javascript/qa-rest-api/node_modules/kareem/index.js:216:48 at /Users/ian/Desktop/icloud/pgm/treehouse/javascript/qa-rest-api/node_modules/kareem/index.js:127:16 at _combinedTickCallback (internal/process/next_tick.js:67:7) at process._tickCallback (internal/process/next_tick.js:98:9) [nodemon] app crashed - waiting for file changes before starting...
1 Answer
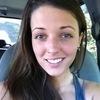
Amber Cyr
19,699 PointsNot sure if you still need the answer, but the closing bracket for Animal.find needs to be after the db.close statement. If it is before, the forEach method will error as undefined.