Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial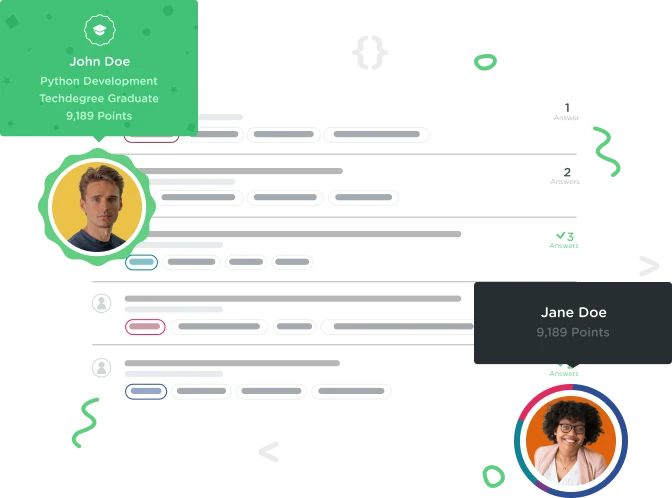

Frank keane
8,074 PointsWhy the extra parentheses?
When you added index parameter you added a bracket, why?
same in MDN docs, suddenly the bracket appears
array1.forEach(element => console.log(element));
forEach((element) => { ... } ) forEach((element, index) => { ... } ) forEach((element, index, array) => { ... } )
Thanks!
2 Answers
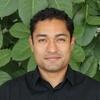
Juan Luna Ramirez
9,038 Pointsdo you mean the curly braces {}
when you say brackets?
- The curly braces are not a result of having the index parameter in your example. The braces are about implicit and explicit returns. Removing the braces allows you to return a value implicitly. More on explicit vs implicit return below.
- As for the parentheses, they are needed if you have no parameters or more than one. They are optional if you only have one.
some example:
// - old school function
// - this function doesn't do anything yet
// - note that although there is no return statement, it will implicitely return
// undefined
const formatName = function(firstName, lastName) {
/* function body here */
}
console.log(formatName()) // will log undefined
// same as above but I'm explicitely returning undefined just to explain the
// difference between implicit and explicity return
const formatName = function(firstName, lastName) {
return undefined
}
console.log(formatName()) // will also log undefined
// - ok, let's do some work inside the function. Simply return a formatted
// version of a person's name.
// - We need to explicityly return a string of the formatted name
const formatName = function(firstName, lastName) {
return `${lastName}, ${firstName}`
}
console.log(formatName('Frank', 'Keane')) // logs "Keane, Frank"
// - now let's do the exact same thing but with an arrow function
// - note that we are still using an explicity return statement
const formatName = (firstName, lastName) => {
return `${lastName}, ${firstName}`
}
console.log(formatName('Frank', 'Keane')) // also logs "Keane, Frank"
// - since this is simple function that can be written in one line, we can take
// advantage of arrow function implicit return by removing the curly braces
// that open and close the body of the function.
// - You would need to keep the curly braces if you needed to more things within
// the body of the function.
const formatName = (firstName, lastName) => `${lastName}, ${firstName}`
console.log(formatName('Frank', 'Keane')) // also logs "Keane, Frank"
// - now a function example with one parameter
// - having the parentheses is valid but optional
// - let's use an implicit return here as well
const sayHello = (name) => `hello ${name}!`
console.log(sayHello('Frank')) // logs "hello Frank!"
// - this is also valid since we only have one parameter
const sayHello = name => `hello ${name}!`
console.log(sayHello('Frank')) // also logs "hello Frank!"
// now a function example with no parameters
// let's use an explicit return for fun
// must have the parentheses
const sayHi = () => {
return 'hi'
}

Frank keane
8,074 PointsThanks for your detailed answer Juan, only seeing it now, thought I had emails set. I get it now, thanks!