Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial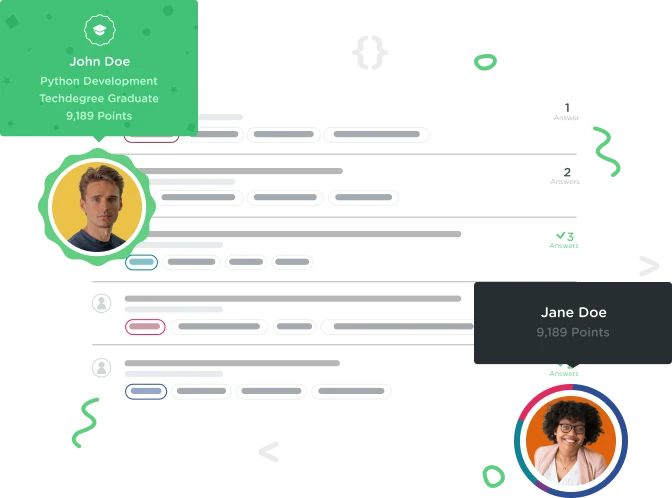

Arun Patel
1,180 PointsWhy the function didn't return right answer?
I executed same code separately and got right answer (25)
def squared(x): try: x = int(x) y = x * x return y except ValueError: x = str(x) z = x + x return z
def test(): x = squared(5) print(x)
test()
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(x):
try:
x = int(x)
y = x * x
return y
except ValueError:
x = str(x)
y = x + x
return y
1 Answer
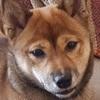
Katie Wood
19,141 PointsHello there,
You're close - the only thing not functioning correctly is when it passes a string value. With the way it works right now, using x + x, it will just print the string twice. However, it is supposed to print the string a number of times equal to the strings length, so it should instead be x * len(x). With that one line changed, it looks like this:
def squared(x):
try:
x = int(x)
y = x * x
return y
except ValueError:
x = str(x)
y = x * len(x)
return y
If you have things spaced out to make it easier to read as you're learning, that's totally fine. If you're interested, though, this can be shortened a good bit:
def squared(x):
try:
return int(x) * int(x) #can also do int(x) ** 2 (exponent notation)
except ValueError:
return x * len(x)

Arun Patel
1,180 PointsThanks a lot for the clarification.
Anibal Marquina
9,523 PointsAnibal Marquina
9,523 Points