Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial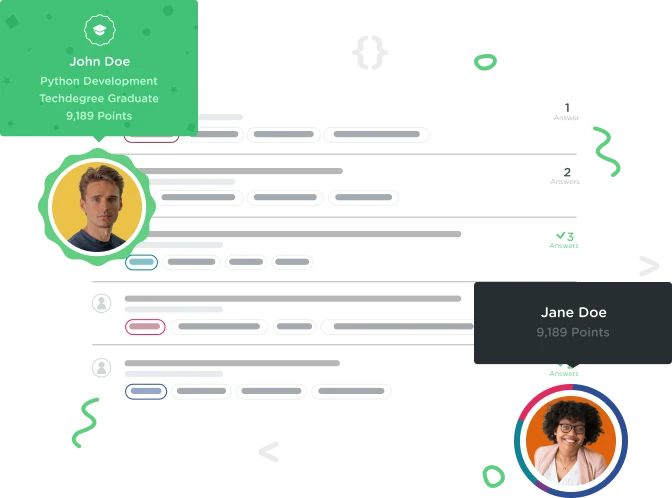

Evan Waterfield
3,240 PointsWhy the syntax error?
import random
# safely make an int
# limit the guesess
# too high nmessage
# to low message
def game():
# generate a radonm number between 1-10
secret_num = random.randint(1, 10)
guesses = []
while len(guesses) < 5:
try:
# get a number guess from the player
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("{} insn't a number!".format(guess))
else:
guesses.append(guess)
# compare guess to secret number
if guess == secret_num:
print("You got it! my number was {}".format(secret_num))
break
elif guess < secrect_num:
print("My number is higher than {}".format(guess))
else:
print("My number is lower than {}".format(guess)
else:
print("You didn't get it! My number was {}".format(secret_num))
play_again = input("do you want to play again? Y/n")
if play_again.lower() !='n':
game()
else:
print("Bye!")
game()```
4 Answers
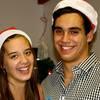
Cody Te Awa
8,820 PointsOkay this problem doesn't wanna let up. Copy and paste this code and tell me if you get any errors: It is just your code with syntax fixes
import random
# safely make an int
# limit the guesess
# too high nmessage
# to low message
def game():
# generate a radonm number between 1-10
secret_num = random.randint(1, 10)
guesses = []
while len(guesses) < 5:
try:
# get a number guess from the player
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("{} insn't a number!".format(guess))
else:
guesses.append(guess)
# compare guess to secret number
if guess == secret_num:
print("You got it! my number was {}".format(secret_num))
break
elif guess < secret_num:
print("My number is higher than {}".format(guess))
else:
print("My number is lower than {}".format(guess))
else:
print("You didn't get it! My number was {}".format(secret_num))
play_again = input("do you want to play again? Y/n")
if play_again.lower() !='n':
game()
else:
print("Bye!")
game()

Evan Waterfield
3,240 PointsOf course it worked! Now I'm just going to compare the two side by side. Things kept getting shifted from me screwing up the format originally then copying and pasting stuff. Thanks for your help I really appreciate it!!! The indentation usually gets me but hey you screw and then you learn.

Evan Waterfield
3,240 PointsOK side by side I found a few things,
else:
guesses.append(guess)
# compare guess to secret number
if guess == secret_num:
print("You got it! my number was {}".format(secret_num))
break
elif guess < secret_num:
print("My number is higher than {}".format(guess))
else:
print("My number is lower than {}".format(guess))
my elif and the else following needed indention and I had a : following my elif. Why doesn't that elif need the : ?
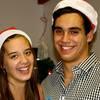
Cody Te Awa
8,820 PointsHi Evan, Although the provided information isn't the cleanest I can spot a few errors in your code that I will let you know of:
"""SYNTAX ERRORS"""
#1st one is possibly the indentation is 8 spaces instead of 4 spaces after the while loop
#2nd one is there is a missing parenthesis on line in your first else statement this one:
print("My number is lower than {}".format(guess)
#3rd one is the fact that there is 2 else statements. You can only have one else
# statement therefore the first one should be a elif or isn't necessary
"""REFERENCE ERRORS"""
#4th one is the spelling of the secret num on this elif condition
elif guess < secrect_num:
Apart from that the code looks great Evan, let me know how you got on after fixing those errors would love to hear from you. Happy Coding Evan! :)

Evan Waterfield
3,240 PointsThanks for the response. I made some changes but still keep coming up with syntax and indention errors. kinda confused at this point since I have made some changes. here's what it looks like now. i get syntax error line 31
import random
# safely make an int
# limit the guesess
# too high nmessage
# to low message
def game():
# generate a radonm number between 1-10
secret_num = random.randint(1, 10)
guesses = []
while len(guesses) < 5:
try:
# get a number guess from the player
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("{} insn't a number!".format(guess))
else:
guesses.append(guess)
# compare guess to secret number
if guess == secret_num:
print("You got it! my number was {}".format(secret_num))
break
elif guess < secret_num:
print("My number is higher than {}".format(guess))
else:
print("My number is lower than {}".format(guess))
else:
print("You didn't get it! My number was {}".format(secret_num))
play_again = input("do you want to play again? Y/n")
if play_again.lower() !='n':
game()
else:
print("Bye!")
game()```
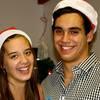
Cody Te Awa
8,820 PointsHi Evan, your code looks great now, there is just a slight indentation error on your elif and else statement on line 31 and line 33. I have tested it in my local environment and I just had to bring those 2 statements back a smidge (They appear to be 2 spaces indented too far) so they were in line with the original if-statement. Hope that helps! Happy coding Evan! :)

Evan Waterfield
3,240 PointsOk I got that part fixed but now I am getting a syntax error on line 39 from the else: ?

Christian Scherer
2,989 PointsGuys,
My try except block doesn't work, giving me the following:
Traceback (most recent call last):
File "numbers_game.py", line 27, in <module>
main()
File "numbers_game.py", line 11, in main
print('{} is not a number, please try again'.format(guess))
UnboundLocalError: local variable 'guess' referenced before assignment
My code is the exact same one everybody else has.
try: guess = int(input('Please enter your number: ')) except ValueError: print('{} is not a number, please try again'.format(guess)) else: .........................
Any idea?
Steven Parker
231,269 PointsSteven Parker
231,269 PointsTo fix the formatting, follow the code instructions in the Markdown Cheatsheet pop-up below the answer area.
Click on the little box with 3 dots in it to expose the option to edit your question. Once you get it fixed, it will be much easier for someone to look into your issue.