Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial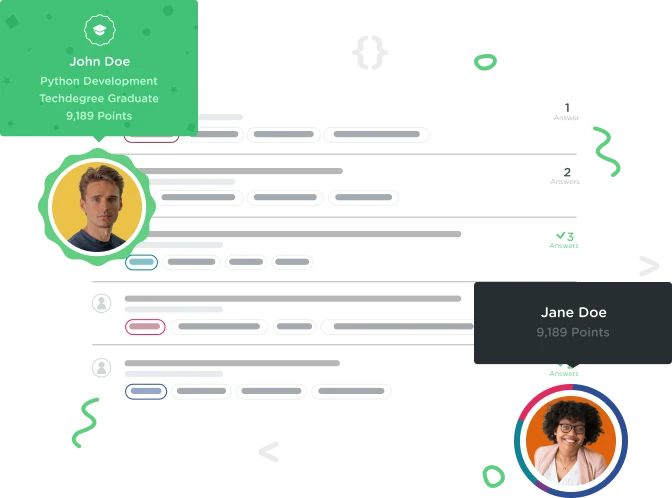
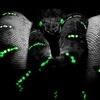
Robert Peters
3,728 PointsWhy the use of books=None?
I'm still working through this video to understand classmethods properly, so I might be missing something that seems obvious, but this use of books=None has stumped me, can anyone explain?
class Book:
def __init__(self, title, author):
self.title = title
self.author = author
def __str__(self):
return '{} by {}'.format(self.title, self.author)
class Bookcase:
def __init__(self, books=None):
self.books = books
@classmethod
def create_bookcase(cls, book_list):
books = []
for title, author in book_list:
books.append(Book(title, author))
return cls(books)
bc = Bookcase.create_bookcase([('Moby-Dick', 'Herman Melville'), ('Jungle Book', 'Rudyard Kipling')])
In the video it says that "you shouldn't use a mutable object as the default argument to an argument", but I'm not exactly sure what that means .
Also, if anyone has the time, could they go through a step-by-step as to what's actually happening when the command is called? I'm struggling to follow what's being passed where!
2 Answers
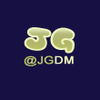
Jonathan Grieve
Treehouse Moderator 91,254 PointsMutable means an object that has values that can change. Something is immutable, im programming terms that means Objects that cannot be changed.
In OOP Python books=None
is simply passing in a default value as a parameter to the init method of the BookCase class. You're assigning a default value to the books parameter on creation of an instance,
So what your code is doing is creating an initial instance with default values, and then creating a new instance that passes in values to your create_bookcase()
method.
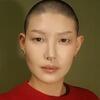
Begana Choi
Courses Plus Student 13,126 PointsI also wonder about this ! :-/ looking forward clear answer! thank you!
Robert Peters
3,728 PointsRobert Peters
3,728 PointsCheers pal! I get that it's mutable, I guess I just don't really know why you shouldn't pass a mutable object as an argument. Up to now we've been passing mutable objects to methods, like lists or dicts. Is there a reason that it isn't just:
or:
I mean, we pass a list in straight away don't we?