Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial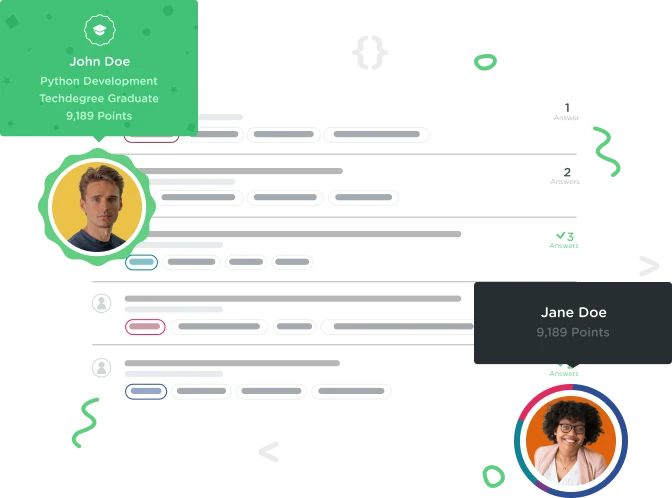

Daniyar Basharov
2,957 PointsWhy there is no ERROR message when I enter NaN as an argument like '100' insteat of 'hunred'
here is my code
function getRandomNumber(bottomNumber, topNumber) {
var betweenRandomNumber = Math.floor( Math.random() * (topNumber - bottomNumber + 1)) + bottomNumber;
if ( isNaN(bottomNumber) === true || isNaN(topNumber) === true ) {
throw new Error("Some arguments are not a number");
} else {
return betweenRandomNumber;
}
}
console.log( getRandomNumber (10, 20) );
console.log( getRandomNumber (2, '100') );
console.log( getRandomNumber (100, 500) );
console.log( getRandomNumber ('200', 300) );
console.log( getRandomNumber (1000, 10000) );
thanx

Mark Bacolcol
7,354 Points@Aaron - A number within a string (isNaN("20")) runs false for me and the function returns an appropriate value. I tried using "20test" as a parameter and received an error as expected. So does JS treat strings with only numbers, as numbers?
Aaron Martone
3,290 PointsAaron Martone
3,290 PointsBecause JS attempts to coerce your value to a data type depending on how you reference it. If you do '100' + 10, JS coerces the 10 number into a string and performs '100' + '10' resulting in '10010'. And '10010' is a String, despite it looking like a number to us. A String is NOT a NaN value. Try dividing 0/0, the result is NaN. So if you console.log( isNaN( 0/0 ) ); you get true. But if you log console.log(isNaN('10010')) you get false, because a String value (even though it looks numeric) is NOT a NaN value.