Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial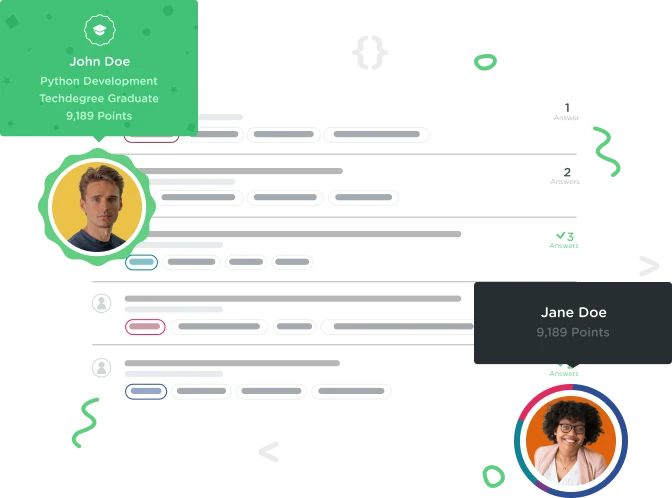

ALBERT QERIMI
49,872 Pointswhy this error?
when I print the word no vowels in it but it says Bummer! Hmm, got back letters I wasn't expecting!. and anybody another way to check vowels for ex "a","A"
vowels = ["a", "e", "i", "o", "u","A","E","I","O","U"]
new_word= []
def disemvowel(word):
for letter in word:
if letter not in vowels:
new_word.append(letter)
else:
continue
word=''.join(new_word)
print(word)
return word
disemvowel("aeioup")
4 Answers
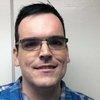
Andrew Calhoun
19,360 Pointsadren beat me too it. The issue stems from using the new_word array list as a global, rather than a locally scoped variable. This is the danger of global scope! It can have highly unexpected and undesirable results. There are times when it is appropriate, but this is a case in point of when it is inappropriate. :)
Chin up though! I have a CS degree from a reputable engineering school and I still make these types of mistakes on a regular basis.
Another way to solve this, if you want to avoid lists, and just go with straight strings and variables is as such and is a bit more 'destructive' than your and adren's solutions:
def disemvowel(word):
vowels = "AEIOUaeiou"
for letter in word:
if letter in vowels:
word = word.replace(letter, "")
print(word)
disemvowel("aeioup")

andren
28,558 PointsThe issue is that the new_word
variable is placed outside the function. That creates an issue if you run the function multiple times. Here is an illustration:
# First call
disemvowel("aeioup")
# This would return "p" which is correct
# Second call
disemvowel("Hello")
# This would return "pHll" which is incorrect. The "p" is a leftover from the first call to the function.
This issue can be fixed by simply moving the new_list
variable inside the function. It is generally considered bad practice to keep variables that belong to a function outside of it, as it pollutes the global namespace in Python. And can lead to bugs like the one that occurred here.
Also as far as checking without having to include both lower and uppercase letters in the list, that can be done by using the lower
function on the letter
when you check if it is present in the vowel
list. Like this:
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u"]
new_word = []
for letter in word:
if letter.lower() not in vowels: # lower the letter as it is being compared to the list
new_word.append(letter)
else:
continue
word=''.join(new_word)
print(word)
return word

ALBERT QERIMI
49,872 PointsThank's mates i understand now (Y)

satish v
1,177 PointsHi ...
can you check this function .. it works perfectly fine for me but still throws an error Hmm, got back letters I wasn't expecting!
def disemvowel(word): lower_vowels = ["a","e","i","o","u"] upper_vowels = ["A","E","I","O","U"] word = list(word) while True: for letter in word: if letter in lower_vowels or letter in upper_vowels: word.remove(letter) word = ''.join(word) return word
Andrew Calhoun
19,360 PointsAndrew Calhoun
19,360 PointsIn addendum, use of .upper()/.lower() helps keep your code a bit cleaner, but I figured I'd show you a 'fully' hand-coded version so you can get an idea of other approaches to this sort of problem as well.