Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial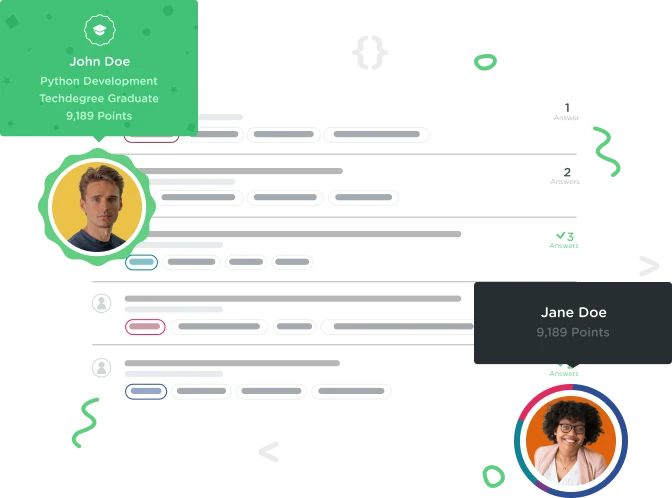

Samar Khanna
1,757 PointsWhy this syntax?
When Amit create an override func, to specify the default value for percentage we had to use
override func discountedPrice(_ percentage: Double = 10.0) -> Double {
return super.discountedPrice(percentage)
}
But when creating a convenience init, the syntax used was:
convenience init(description: String) {
self.init(description:description, price: 99, designer: "Calvin Klein")
}
Why didn't we use an underscore, or an equal to sign?
3 Answers
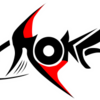
Justin Black
24,793 PointsI've just gone through this video and while my verbage may not be 100% here is the gist of why the different syntaxes.
The first example:
override func discountedPrice(_ percentage: Double = 10.0) -> Double {
return super.discountedPrice(percentage)
}
We use override here, as this is a custom method within the super class. The purpose of the _ is for synthesizing, in that our new method, while still functionally identical to that of the superclass, has extra functionality, in this case being the extra functionality being that percentage is an optional.
The second example:
convenience init(description: String) {
self.init(description:description, price: 99, designer: "Calvin Klein")
}
Think of init as more of a system method than anything else. We aren't technically overriding the parent function, more so giving the application the ability to do some of the work for us. In this example we have a store with products, and we only carry products by CK and each item costs 99 dollars. So instead of having to do:
var tshirt = Clothing(title: "product", price: 99, designer: "Calvin Klein")
for multiple products, all we need to pass in is the title, which calls this new convenience initializer, which in turn calls the main designated initializer within the same class, which in turn calls the designated initializer from the super class. So this would be more of a cascading initialization rather than a full on blatant overriding of the init functionality.

Samar Khanna
1,757 PointsSo these are syntax rules? Thanks again!
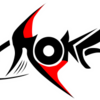
Justin Black
24,793 PointsYes, these are syntax rules.
When you are overriding a method from your super class, you use the override keyword. The entire declaration of this method ( including parameters that it takes ) has to be the same as in the original. Doing what we did, we allow for the use of an optional.
When initializing an instance of a class, you can use the pasthru method, and convenience methods to handle any default settings you may need to pass through that are the same upon multiple types..
Take this example ( ported from what i am working on in ruby right now )
Class User {
let type: String
let username: String
init (username:String, type:String) {
self.type = type
self.username = username
}
}
class Admin:User {
init (username:String, type:String) {
super.init(username: username, type: type)
}
convenience init(username:String) {
self.init(username: username, type: "Admin")
}
}
class General:User {
init (username:String, type:String) {
super.init(username: username, type: type)
}
convenience init(username:String) {
self.init(username: username, type: "General")
}
}

Samar Khanna
1,757 Pointsohhhh. Thanks!!! I got it
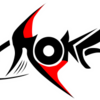
Justin Black
24,793 PointsHappy to help out. Glad you understand it better now. With a single pair of classes it can be hidden as to why and how it would be useful, its not until you can see it in a larger scale that it truly becomes apparent. Have your "AH-HA!" moment?
Samar Khanna
1,757 PointsSamar Khanna
1,757 PointsBut in both examples we are using default values so why does one have an underscore and '=' sign while the other doesn't? Also why don't we have to specify the type in the convenience init? I didn't get that part. Thanks for your help :)
Justin Black
24,793 PointsJustin Black
24,793 PointsThe override method we are using default values in a different way than the init method. In the override, our super class method doesn't have a default value, so we have to modify the functionality of the input to allow for an optional parameter.
The second one we don't, specifically because we're directly calling pass through methods from the top down in a cascading style to provide each init functions desired parameters.
With the first one, we can completely omit sending any parameters and it still functions as our default is 10.
In the other one, we expect the user to submit a single parameter, and then we use passthru to complete our 'default' values to the final destination which is the super class init method.
We can run: ** tshirt.discountedPrice()** but we can't run var tshirt = Clothing() as the second one requires the description parameter, and our 'store' knows that the cost of this is going to be 99 dollars, and be a product of calvin klein.. So, it'll call it like this:
Which, the end result is we have a fully instantiated object with all the necessary elements. This is part of object instantiation and object inheritance. It can be confusing, not only in swift, but once you understand it you'll get that "AH-HA!" moment.