Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial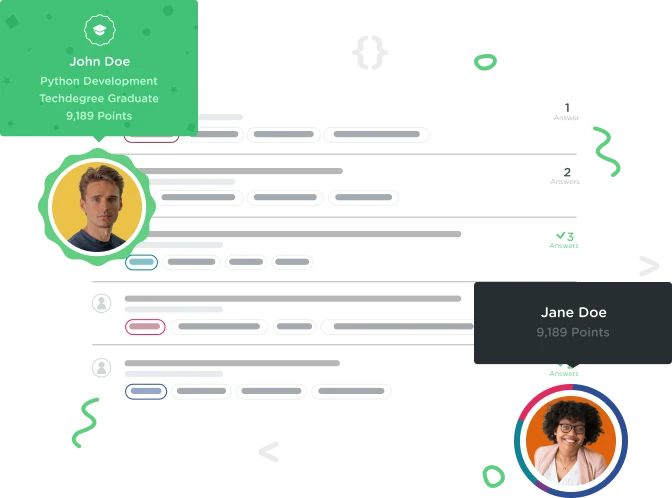

anmo20
6,470 PointsWhy use a loop for switchPlayers?
Couldn't I just write an IF statement like this? I'm not very familiar with For...Of loops so I didn't like her solution because I don't understand it.
switchPlayers () {
if (this.players[0].active === true) {
this.players[0].active = false;
this.players[1].active = true;
} else {
this.players[0].active = true;
this.players[1].active = false;
}
}
1 Answer
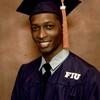
Dane Parchment
Treehouse Moderator 11,076 PointsOkay, so let's break this down so that it's easier to understand why she used the solution she used. I'll preface this by saying it's really good that you are thinking like this and asking these questions, it's how you grow as a developer. I say that because I'm about to tear into your code.
So let's start with what's wrong with your solution:
switchPlayers () {
if (this.players[0].active === true) {
this.players[0].active = false;
this.players[1].active = true;
} else {
this.players[0].active = true;
this.players[1].active = false;
}
}
-
You misunderstood what the point of the
switchPlayers()
function is. I'll admit, it's a terribly named function, since you would think that it is supposed to just switch who the currently active player is. However, this is what the actual prompt for this function is:Inside the method, iterate through the array of Players (Look closely at the Game class constructor method if you need a reminder on how to access the Players). For each Player object, switch the value of its active property. If the active property is set to true, it should now be set to false and vice versa.
Notice how we are supposed to go through each player and switch their active status. Not switch who is the active player, which is what your code is doing. - Let's assume that your code was the correct way to switch who the active player is. This solution will only work on a game that has exactly two players. But what if the game needed more than two players? Your solution isn't scalable because for each new player added we'd need to account for 2^n different combinations of player active states. For example if there were 3 players you need to write 7 - 8 different if statements to account for all the possible combinations of active players.
So basically for starters your code isn't actually doing what the instructor is asking you to do, and even if it was, it wouldn't be very scalable for more than 2 players.
So let's look at what the instructor did, and explain why it works.
switchPlayers() {
for(let player of this.players) {
player.active = player.active === true ? true : false : true;
}
}
Okay so let's start with the loop. A for...of
loop is a special type of loop in javascript that is meant to iterate over iterable objects. Basically a data structure that implements an Iterable (don't worry about this for now) and an array is one such data structure.
So basically the syntax for the for...of loop looks like so: for(something of somethingelse)
basically. The somethingelse
is the data structure we are iterating over, and the something
is the current element that we have iterated on. A quick example:
let arrayOfNums = [1, 2, 3];
for(let n of arrayOfNums) {
console.log(n);
}
This would print to the console: 1 2 3
Now that we know how the for...of loop works. Let's look at what she is doing.
player.active = player.active === true ? true : false : true;
basically she's looping through each player in the array and literally just flipping the active status.
She does this through a ternary statement (shorthand if-statement), which translates to:
if(player.active) {
player.active = false;
} else {
player.active = true;
}
So in short, she is making a scalable solution that loops through each of the players and flips their active state.
anmo20
6,470 Pointsanmo20
6,470 PointsThanks for explaining it this way. I understand better how that loop works, and why it would be better for a more scalable game.
In this specific use case, this game will never have more than 2 players, so while the IF statement I wrote doesn't explicitly do what she was asking, it still has the same outcome of switching which player is active and would provide the same results in this specific case, wouldn't it?
Dane Parchment
Treehouse Moderator 11,076 PointsDane Parchment
Treehouse Moderator 11,076 PointsIn this specific use case it would depend on whether or not both players can be active at the same time. In which case your code wouldn't work because if both players are active then only one of the players will have their active state flipped.
It's good to get into the habit of following the functional requirements of your code. Because while your code, could technically solve the problem if both players cannot be active at the same time. It's not actually doing what the functional requirements asked you to do.
So while your solution may indeed work. If later it's decided that their should be more than one player, or that multiple players can be active at the same time, your code would require a re-work, while her's would not.
anmo20
6,470 Pointsanmo20
6,470 PointsRight, I understand. I tested my code after getting the game to a finished state to see if it works, and it does.
But I completely understand that despite accomplishing the same outcome, it's not actually doing the right thing.
Thanks for your explanation!