Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial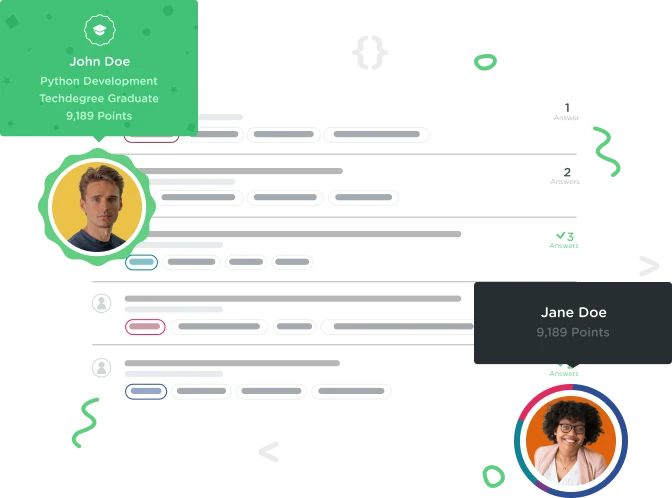
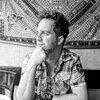
jlampstack
23,932 PointsWhy use {} curly braces for return inside setState() ?
Please help me understand.
When we use the return inside the render() {} we use parenthesis
render() {
return (
// React Elements and HTML Elements go here
);
}
So why are we now using {} curly braces inside our setState()
incrementScore = () => {
this.setState( prevState => {
return {
score: prevState.score += 1
}
});
}
2 Answers
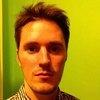
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsThe render
method should return JSX. You don't have to use parens (), but if you want your JSX to take up multiple lines, it's handy.
We can do this:
render() {
return <div>This is fine</div>
}
But we can't do this:
render() {
return
<div>
<p>This is not fine.</p>
</div>
}
...because we'll just end up returning nothing and it will ignore the line after return.
We can do this:
render() {
return <div>
<p>This is okay.</p>
</div>
}
... and it's is fine with returning the multiline JSX that was started on the same line as return
. So we don't need parens, but some people say it's cleaner and easier to read to wrap it in parens, and there's an eslint rule for it if you're using eslint (I highly recommend).
render() {
return (
<div>
<p>This is fine too.</p>
</div>
)
}
We also can't do this, because now we're returning an object with some JSX in a way that it can't parse. It doesn't want an object:
render() {
return {
<div>
<p>This will break.</p>
</div>
}
}
Okay onto setState()
. So setState can take one of two things, an object, or a function that returns an object.
This version in your question is a function that returns an object:
this.setState( prevState => {
return {
score: prevState.score += 1
}
});
Or we could do it the way where we just return an object:
this.setState({ score: this.state.score + 1 });
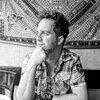
jlampstack
23,932 PointsThanks Brendan Whiting for taking the time to explain in detail. It seems so simple now. There are so many different ways of doing the same thing that it's easy for a noob like myself to get tripped up. I was overthinking it.
It was more the setState() that was tripping me up. To clarify you explanation, we can still use parens () for the return inside of setState()
but they are optional and probably easier and cleaner to read without the parens ()
// Code still works with parens () but adds more clutter
this.setState( prevState => {
return ({
score: prevState.score += 1
})
});
// Code without parens() is cleaner / easier to read
this.setState( prevState => {
return {
score: prevState.score += 1
}
});
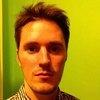
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsThat's correct. In JavaScript, you can basically wrap any expression in parentheses and it doesn't change that expression, it's just a handy wrapper for multiline expressions or even just readability. It's kind of like how in algebra we can wrap things in parentheses in order to make the order of operations work a certain way or to be more clear: (1 + 8) / 3
.
jlampstack
23,932 Pointsjlampstack
23,932 PointsBrendan Whiting can you answer one more question? Why do we need a return inside or setState() ?
We didn't need it before.
Brendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsBrendan Whiting
Front End Web Development Techdegree Graduate 84,738 PointsThere are two ways of calling
setState
, you can pass in an object, or you can pass in a function. If you pass in a function, that function needs to return an object.