Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial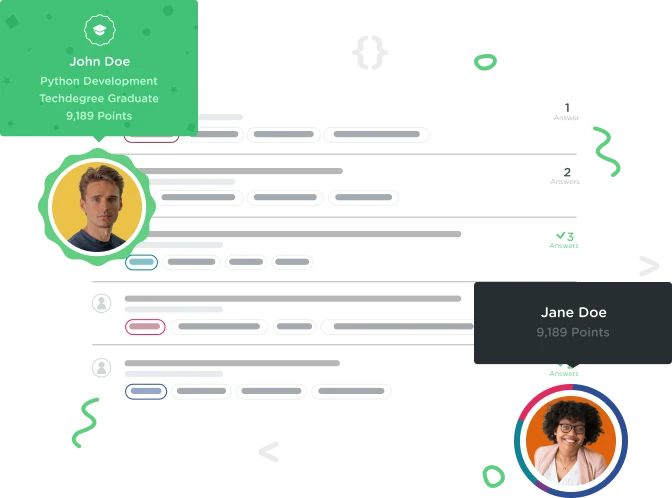

Nataliya Fateeva
Python Development Techdegree Student 4,858 PointsWhy use datetime instead of string concatenation for Wiki Challenge?
It seems that "ask for date input/generate wiki link" challenge could be done easier by just concatenation of user input strings, since URL is basically a string (I am omitting all catch-error/loop parts):
month = input("Please type in month in EN (e.g. June) > ") month = month.capitalize() day = input("Please input day of the month as integer > ") final_date = f'{month}_{day}' wiki_format = f'https://en.wikipedia.org/wiki/{final_date}' print(wiki_format)
As we already accepted that we are not handling language-agnostic case (by hard-coding en link in the offered solution), it seems this is not a good case that actually needs strptime()/strftime() methods and using strings is simpler..
What am I missing?
2 Answers
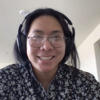
AJ Tran
Treehouse TeacherHi Nataliya Fateeva!
The beauty of programming is that there are many ways to do things! You make a valid point -- that this exercise can be completed with string concatenation. I know that the example is quite "contrived" -- it exists to introduce a new concept so that you can get some experience with using the datetime
module.
In the "big picture," this is just one introduction to working with datetime
and it will most certainly not be the last time that you will work with dates and times in your coding journey! There are also modules for calendar
and time
!!!
For example, when working with Databases, records are often saved with a timestamp that is hard to read for humans. It's a very common practice to convert those weird datetime
into a "friendlier" string for humans to read.
You benefit now by recognizing that 1) Python has a built-in module for datetime
and 2) you know specifically how to use methods such as strptime
and strftime
.
One BIG thing about these modules is that there is a lot of "under the hood" code that will seriously help you out, like with validating data. Hypothetically, what would happen in your program if a user gave you a non-real dates like "January 44" or "March -2"?
>>> import datetime
>>> datetime.date(2021, 1, -1)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: day is out of range for month
How about keeping track of Leap Years, when February has one extra day? (2/29).
>>> datetime.date(2020, 2, 29)
datetime.date(2020, 2, 29)
>>> datetime.date(2021, 2, 29)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ValueError: day is out of range for month
These modules exist because they have all the code written to handle very common patterns that countless programmers have been dealing with since... the beginning of programming! Having a greater understanding of what is all part of the Python Standard Library will make you well-equipped :)
I hope this helps you!

Nataliya Fateeva
Python Development Techdegree Student 4,858 PointsThank you for the detailed response! I guess it was just me wishing for an exercise that would show case the usefulness of datetime's strptime/strftime methods in a more straight-forward way:)
cheers!:)