Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial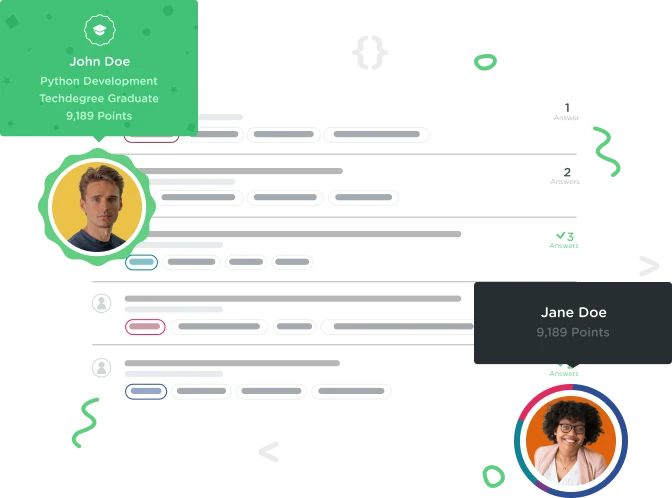

Bryan Guillen
1,051 PointsWhy use instance variables what is a point? Also, why set that instance variable to the same thing without the @ symbol
Hey,
Going through Ruby Objects and Classes.
I get why we use objects and classes. However, I do not get why we use instance variables? What is the function of an instance variable? Why do we set instance variables to the same word?
For example: @name = name....
Why this?
1 Answer
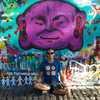
Andrew Stelmach
12,583 PointsNot sure exactly what you mean by your example - I'd have to see the full code. However, instance variables have a number of uses. The main benefit of instance variables is to do with scope.
class MyClass
def method1
local_variable = "hello"
end
def method2
puts local_variable
end
end
my_class = MyClass.new #creates an instance of MyClass and assigns it to a variable, my_class
my_class.method1 #assign the string 'hello' to a local variable, local_variable.
my_class.method2
=> NameError: undefined local variable or method `local_variable' for MyClass:0x00000001581cf8
local_variable 's scope is limited to method1 - it is only accessible from inside method1
class MyClass
def method1
@instance_variable = "hello"
end
def method2
puts @instance_variable
end
end
my_class = MyClass.new
my_class.method1
my_class.method2
hello #'puts' prints the value of the instance variable, @instance_variable, and then:
=> nil #returns nil ('puts' always returns nil).
@instance_variable is accessible from inside method2.
This is just one example of an instance variable's scope.
A common use of instance variables in Ruby is to use them inside a class's initialize method, along with an attr_reader, to allow something like:
some_class = SomeClass.new
some_class.some_instance_variable
=> "a value"
For example:
class MyIdentity
attr_reader :source
def initialize
@source = "Primarily my childhood and later shaped by hedonistic experiences and time abroad doing a lot of cool and crazy shit"
end
end
my_identity = MyIdentity.new #when the class is instantiated, the initialize method is run automatically.
my_identity.source
=> "Primarily my childhood and later shaped by hedonistic experiences and time abroad doing a lot of cool and crazy shit"
For further reading, check this out, and for an explanation of attr_reader (and also check out attr_writer and attr_accessor), Google is your friend ;-)
Bryan Guillen
1,051 PointsBryan Guillen
1,051 Pointsyeah google definitely is my friend... Your answer really helped... It's just different when you have no references to a brand new world like 'the world of programming'. so it is always great to have people like you especially on this website. Thanks.