Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial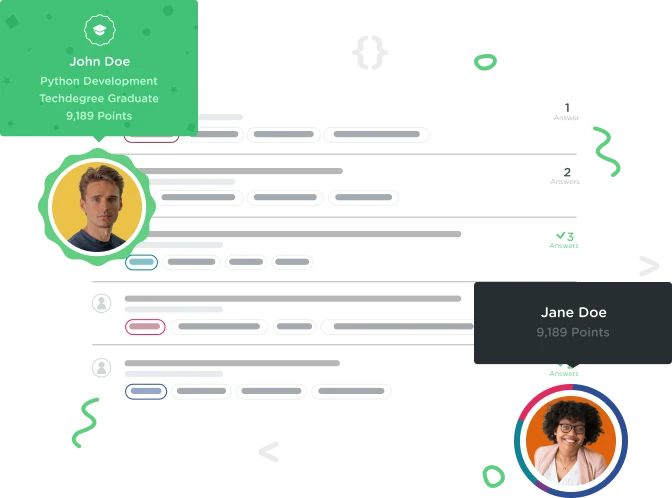

Liz Karaffa
17,576 PointsWhy Use jQuery for a Mobile Drop Down
I'm glad we did this stage because it was good to learn all the things it taught and to change HTML with jQuery.
My question is more philosophical in nature. If the whole point is to give the user a better experience on a mobile device, why would we approach this from jQuery instead of just doing media queries in CSS from the get go and then have the one jQuery event where we do this
//Bind change listener to the select
$select.change(function(){
//Go to select's location
window.location = $select.val();
});
Since we've been told to be as unobtrusive as possible, if a mobile device has shodding internet connection or javascript disabled, why would we want the entire experience to hinge on this code being able to run?
2 Answers
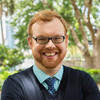
Andrew Chalkley
Treehouse Guest TeacherGood question! Asking these types of questions help find better solutions. But each solution comes with it's own unique set of problems.
Let's talk about the problems with the code from the current project:
OK so let's take our code we just did in the stage. If we ever alter a link in the menu it will automatically be added to the drop down. So you're not modifying the same functionality in two places. One could forget to add the link in a drop down if it wasn't generated automagically by the jQuery. If the menus were generated by some sort of server-side language we may not need to worry about that. Since this is plain old HTML we do.
OK so if the jQuery doesn't kick in the media query hides the links which is bad...because there's not even the links in the menu displaying on smaller browser widths.
To mitigate this problem we could add a class like:
$("#menu").addClass("js_present").append($select); //Line 6 of app.js
And then alter the media queries like so:
@media (min-width: 320px) and (max-width: 568px) {
#menu.js_present ul {
display:none;
}
}
@media (min-width: 568px) {
#menu.js_present select, #menu.js_present button {
display:none;
}
}
We could style the menu (unordered list and list items) better for when JavaScript is disabled so it does look less "gross".
/**
Styling when JavaScript is disabled.
**/
@media (min-width: 320px) and (max-width: 568px) {
#menu ul li {
width:15%;
font-size:12px;
}
}
/**
Modify CSS to hide links on small width and show button and select
Also hides select and button on larger width and show's links.
Styling when JavaScript is enabled.
**/
@media (min-width: 320px) and (max-width: 568px) {
#menu.js_present ul {
display:none;
}
}
@media (min-width: 568px) {
#menu.js_present select, #menu.js_present button {
display:none;
}
}
That would probably be the best solution. So the user can still get to the pages when their connection / device is flakey.
However to respond to your specific question - "Since we've been told to be as unobtrusive as possible, if a mobile device has shodding internet connection or javascript disabled, why would we want the entire experience to hinge on this code being able to run?"
The whole point is to enhance the experience. So given the improvements above - they still can get to the content. That's all that's required. Anything else is superfluous. We're progressively enhancing it with the jQuery code.
Now we have a more sensible default, but we also enhance it with jQuery. Tapping little links on a mobile device may be a bit tricky but at least they can get there. Having the drop down menu enables us to get the user's intent better when navigating.
Does that clear things up?

Liz Karaffa
17,576 PointsThat makes a lot of sense about not repeating code for the drop down menu versus the tab-like menu. Trying to keep things DRY. With the default code that you just mentioned above in place in case the jQuery doesn't run, the drop down enhancement makes perfect sense. Thanks for the in depth answer!

James Barnett
39,199 PointsProgressive enhancement for the win.