Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial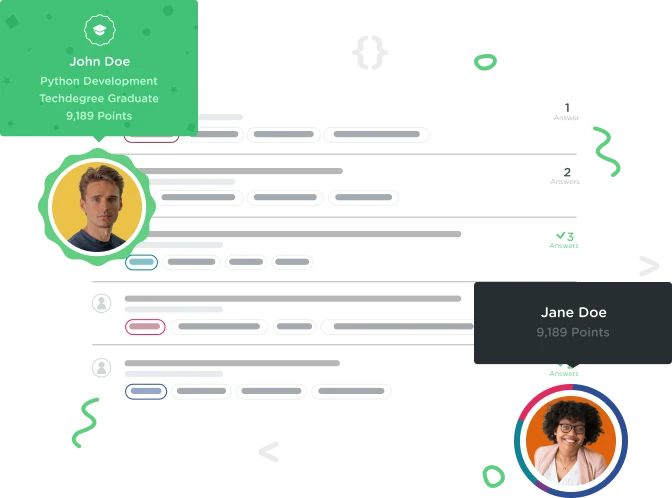

christill
6,500 PointsWhy use map?
What is the benefit of using the map function to toggle the state over something like the below?
this.setState({
guests[index]: !this.state.confirmed
});
2 Answers
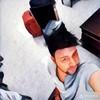
Ari Misha
19,323 PointsHiya Chris! Map function is really flexible and the best thing about it is , it doesnt mutate the original array.Plus the result will be an array as well. You can loop over an array as well in a single statement without using loops. This can come really handy in React. These are the benefits of using Map function.
Now regarding your code , using ! (bang) flips the result, right? In the code , you're setting the current state. And passing in an object containing guests array. Now for current guest , you are flipping the confirmation state with !.
~ Ari
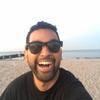
Anthony Albertorio
22,624 PointsI don't see a need to use map either. You have the index already. The index provided by the app won't be out of range, since its limited to the UI on the screen. So, code provided looks unnecessarily complex. Why not just do: Kudos to Kye Buffery for the idea. See: https://teamtreehouse.com/community/can-i-change-directly-the-specific-guest
Also direct mutations to state are not a good idea. The whole idea of the way React is designed is to make thinking about state easy by making it one way and simple.
Like Kye said, see: https://reactjs.org/tutorial/tutorial.html#why-immutability-is-important
toggleConfirmationAt = indexToChange => {
const guestsArr = this.state.guests.slice(); // don't mutate, get copy
guestsArr[indexToChange].isConfirmed = !guestsArr[indexToChange].isConfirmed;
this.setState({guests: guestsArr});
};
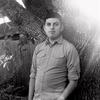
Oziel Perez
61,321 PointsAgreed! I was having some difficulty wrapping my head about this pattern of development, but it makes so much more sense now. By doing this you can more easily track changes in data and you can make unit testing much easier. I realized a while back that I had to get into the habit of writing pure functions in web apps, so I started doing so, but I was still wondering how to apply that to changes in the DOM and to sets of data that would normally exist in a global scope. I now understand that making changes directly to data sets is not as effective as copying the objects first and then loading them back in; something I still hadn't done in my pure functions. React is the framework I didn't know I needed!