Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial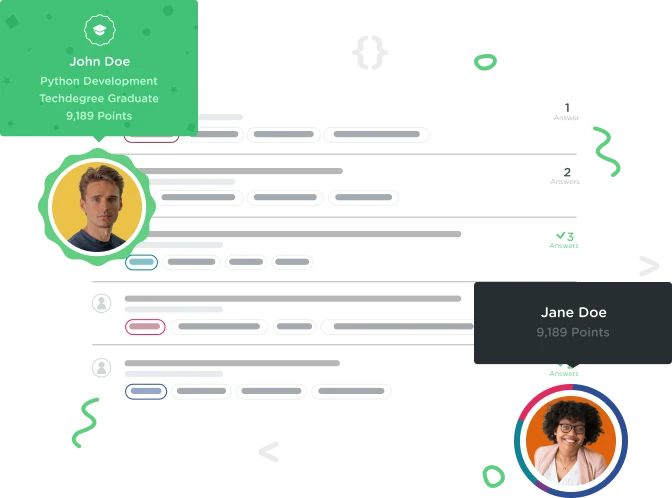
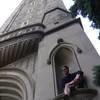
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsWhy use map() when you could just use forEach()?
Guil uses map()
in the generateHTML
function, but map returns a new array. Why doesn't he just use forEach()
which simply iterates over each item in the array? Am I missing something?
3 Answers
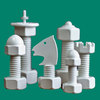
Steven Parker
241,809 PointsIf you used "forEach", you'd need to first create a new empty array, and then inside the body of the forEach you would push the current item onto the new array.
Using "map" saves you a couple of steps since it's designed to return a new array.

Daoud Merchant
5,135 PointsFor anyone else here, I believe .forEach()
to be a better implementation than .map()
(with respect to Guil), since he is appending the values to the DOM within the callback.
-
.forEach()
is best to perform a task with each item of the array in turn (as per this exercise) -
.map()
is best to return a new array where each index contains a value which is some modification of the value at the same index of the original array.
Quick example:
const array = [1, 2, 3, 4, 5];
const doubledArray = array.map(num => num * 2); // [2, 4, 6, 8, 10];
let total = 0;
doubledArray.forEach(num => total += num);
console.log(total); // 30;
// Note: this would be better achieved with '.reduce()', but that's for another time ;)
In my opinion, a more appropriate use of .map()
for this lesson would be something like:
function generateHTML(data) {
const sectionsHTML = data.map(person => `
<div class="section">
<img src=${person.thumbnail.source}/>
<h2>${person.title}</h2>
<p>${person.description}</p>
<p>${person.extract}</p>
</div>`) // Now each person object has been 'mapped' over with a HTML block distributing its data
.join(''); // Remove commas between each
peopleList.innerHTML = sectionsHTML; // Only one write to the DOM :)
};
I'm not saying this is the best way of doing it, but I understand this to be a better usage of .map()
.

JASON LEE
17,352 PointsMy thoughts were the same. The purpose was to iterate over each array and 'do something... period', instead of iterate over each array to return a new modified array.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsYeah but in the video he didnt need a new array. He just needed to iterate over each object in the array and generate html for it and that's it.
Steven Parker
241,809 PointsSteven Parker
241,809 PointsYou didn't give a time index, but I hunted down the part you are referring to — and yes, a "forEach" should work just fine there.