Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial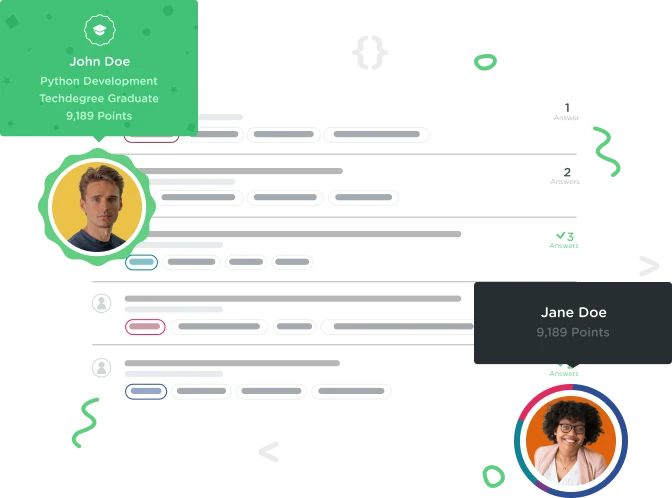
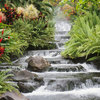
kabir k
Courses Plus Student 18,036 PointsWhy use pure JavaScript to make AJAX requests?
Since jQuery makes AJAX requests faster and easier, why would anyone want to use pure JavaScript to make AJAX requests?
What are the benefits, if any, for using pure JavaScript over jQuery, especially in this instance?
Here are code examples for both:
AJAX request (using pure JavaScript):
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with JavaScript</title>
<link href='http://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<script>
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
document.getElementById('ajax').innerHTML = xhr.responseText;
}
};
xhr.open('GET', 'sidebar.html');
function sendAJAX() {
xhr.send( );
document.getElementById('load').style.display = 'none';
}
</script>
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<div class="heading">
<h1>Bring on the AJAX</h1>
</div>
<button id="load" onclick="sendAJAX()" class="button">Bring it!</button>
<div id="ajax">
</div>
</div>
</div>
</div>
</div>
</body>
</html>
AJAX request (using jQuery):
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with JavaScript</title>
<link href='http://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<script src="//code.jquery.com/jquery-1.11.3.min.js"></script>
<script>
function sendAJAX() {
$('#ajax').load('sidebar.html');
$('#load').hide();
}
</script>
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<div class="heading">
<h1>Bring on the AJAX</h1>
</div>
<button id="load" onclick="sendAJAX()" class="button">Bring it!</button>
<div id="ajax">
</div>
</div>
</div>
</div>
</div>
</body>
</html>
2 Answers
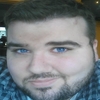
Marcus Parsons
15,719 PointsHi Kabir,
Dave doesn't actually say that it's faster per se to use jQuery all in all. It is faster and simpler to type it, to be sure, but it certainly isn't a faster operation to run the jQuery AJAX commands over pure JavaScript. When using jQuery, the compiler has to go out to the jQuery library, find the functions, and then access any default functions/methods/etc. and execute code accordingly. When using pure JavaScript, the compiler only has to find the default functions/methods/etc. and execute code accordingly.
A developer might want to run AJAX commands without having to depend on an external library like jQuery. If you're grabbing the jQuery from a CDN and that CDN goes down, you've got to find another CDN and then update each instance of linking jQuery. You can probably imagine a headache from this.
With that being said, jQuery is a great library, but there are definitely good reasons why people choose to use pure JavaScript over jQuery.

Erika Suzuki
20,299 PointsHi kabir k ,
I'd prefer to write my AJAX request, event listeners, and DOM manipulation in jQuery. So I could do more with less code. There are no downsides to it if you're gonna use it for AJAX.