Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial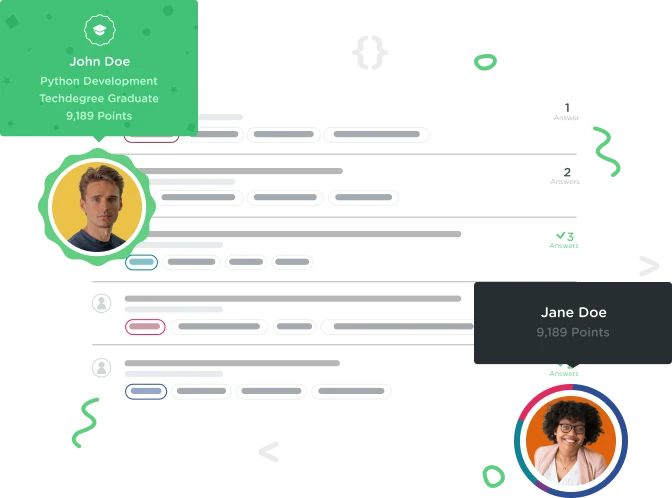

Roland Cedo
21,261 PointsWhy use symbols instead of string keys in this case?
So Jason creates the class "Monster", initializes it with an instance variable called "actions" that is a hash with the key "scream" and value 0.
class Monster
attr_reader :name, :actions
def initialize(name)
@name = name
@actions = {
scream: 0
}
end
def say_something(&block)
block.call
end
def scream(&block)
actions[:scream] += 1
print "#{name} screams!"
yield()
end
end
Now, in the method "scream", he has to access the instance variable "scream" as a symbol so he can increment the instance variable's value:
def scream(&block)
actions[:scream] += 1
print "#{name} screams!"
yield()
end
Can someone clarify why he chose to create "scream" as a symbol instead of a string key/value pairing like:
@actions = { "scream" => 0}
It is currently my understanding that you should use symbols when you want to have an immutable value in your object, correct? If so why use a symbol when we want to increment the value of scream when we call the method "scream"?
1 Answer

rowend rowend
2,926 PointsHi. He uses a symbol because it is more efficient in memory. Side note: when you use symbols inside of a hash you can change the values.