Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial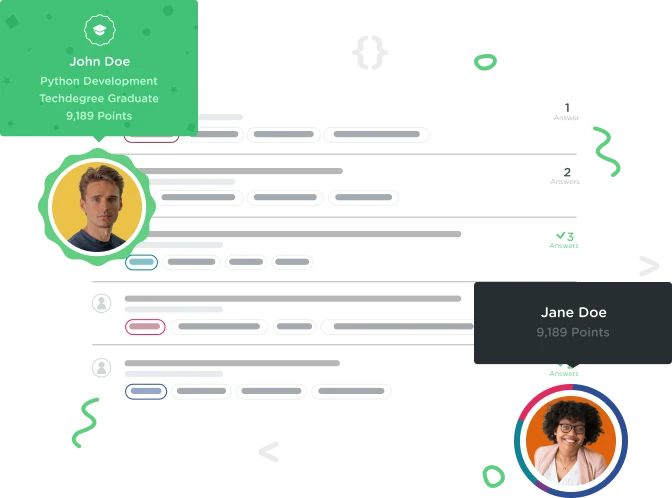
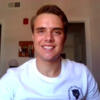
Evan Welch
1,815 PointsWhy use the ".Message" ?
I understand using the variable "ex" out of convention and that you can name variables whatever you choose, however why must we tack on the .Message to the ex.Message? This is the first time I've seen .Message.
Thank you!
4 Answers

Simon Coates
8,223 PointsIt's "dot notation". The variable name is ex. The "Message" is a name of a property on the object. To access the contents of the object 'ex', you need to have fixed names to the innards (methods, properties, fields etc) of ex.
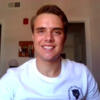
Evan Welch
1,815 PointsThe ex.Message comes up at the bottom part of this code.
using System;
namespace TreehouseDefense
{
class Game
{
public static void Main()
{
Map map = new Map(8, 5);
try
{
MapLocation mapLocation = new MapLocation(20, 20, map);
}
catch(Exception ex)
{
Console.WriteLine(ex.Message);
}
}
}
And the "message" is found where the "throw new OutOfBoundsException" has a string.
namespace TreehouseDefense
{
class MapLocation : Point
{
public MapLocation(int x, int y, Map map): base(x, y)
{
if (!map.OnMap(this))
{
throw new OutOfBoundsException(x + " , " + y + " is outside the boundaries of the map.");
}
}
}
}
What I don't understand is that we never assigned this string concatenation to the word Message so how does the computer know what we are referring to?

Simon Coates
8,223 Pointsoh, with a lot of Exception or Error classes (and subclasses) in different languages, whatever you pass into the Exception constructor will be assigned to a Message (or similarly named) field or property.
using System;
class MainClass {
public static void Main (string[] args) {
try {
throw new Exception("some "+"concatenated "+" string");
} catch(Exception ex)
{
System.Console.WriteLine(ex.Message);//outputs "some concatenated string"
}
}
}
It's just part of the class's established interface and shows up on documentation. (https://docs.microsoft.com/en-us/dotnet/api/system.exception?view=netcore-3.1#properties ). Unless you look at the source code for the Exception class, you're probably not going to see the code that sets the property. You mostly just need to know that it exists. Your interactions with the value will be via dot notation or via Exception constructors - standard or custom. For reference, I'll include a custom exception to demo that you don't really need to know much about it.
using System;
class MainClass {
public static void Main (string[] args) {
Console.WriteLine ("Hello World");
try {
throw new CustomException("some "+"concatenated "+" string");
} catch(Exception ex)
{
System.Console.WriteLine(ex.Message);//outputs "some concatenated string (custom)"
}
}
}
class CustomException: Exception{
public CustomException(String msg): base(msg+" (custom)")
{
}
}
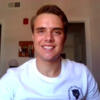
Evan Welch
1,815 PointsThanks a lot Simon. I'll remember that there's a assumed Message field in the Exception class, and that you can write a custom argument when you reference Exceptions, and they will be stored under "Message" unless you declare something like "msg."

Simon Coates
8,223 PointsAn exception should really always have a value for message. And in the demo, the msg parameter was passed into the Message property via the base() call. I only called it msg to demonstrate that the name doesn't matter in that context. You put the value in via the constructor to set it and you access it via .Message.
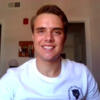
Evan Welch
1,815 PointsGotchu! Thank you for clarifying. Exception messages are always taken as arguments and they are always accessed using .Message.