Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial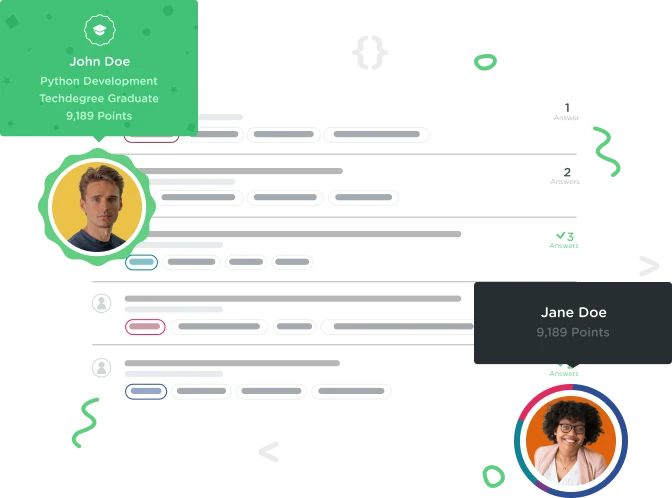

vamosrope14
14,932 PointsWhy was he allowed to put json as a parameter in the annoymous function, if the callback parameter didn't have one?
.
2 Answers
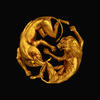
jonathanj
UX Design Techdegree Student 9,378 PointsAmandeep Pasricha I believe what you asking is more to do with what is going on with the lowercase json being thrown in an argument in the callback function within the addEventListener parent function for when you click on the button. It confused me as well until i went back and looked at what all parts make up the getJSON function back before it was being used as a callback function. So if you look at the getJSON function when it was declared he setup the getJSON function so that the 2nd argument is a callback function that in the body of the getJSON function setups up that callback function to have it's own argument which is the data you received once the getJSON gets the data than parses it for you and finally stores it in a variable called data. When the data variable is set up as the getJSON callback function's parameter (aka place holder) it is right there where you can see that the reason why he is able to just drop any random name in place of data when he uses the lowercase json as an argument where he is using getJSON function as a callback for the addEventListener.
Continued blessings, Jonathan
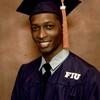
Dane Parchment
Treehouse Moderator 11,075 PointsI am going to make an assumption that you mean, why does the function call for the callback has a parameter when the parameter for the callback itself doesn't contain it.
As in why is this a thing:
function doSomething(text, callback) {
if(typeof callback === function) {
callback('Doing something with ' + text);
}
}
// called like
doSomething("stuff", console.log); // ---> Console logs: "Doing something with stuff."
The parameter callback
is just that, a parameter, it will simply reference whatever you put in it. So we just enter the function, because that is what we are referencing. This allows us to actually call the callback
parameter.
If we were to actually call the function in the parameter, like so:
function doSomething(text, callback(text)) {
if(typeof callback === function) {
callback('Doing something with ' + text);
}
}
it would actually fail for 2 reasons.
Reason 1: The call for the console.log doesn't actually return a function, so this line of code: callback('Doing something with ' + text);
would actually fail, because it would be like doing this: console.log(text)()
which would fail.
Reason 2: Which is the conceptual reason and why reason 1 occurs, is because if you call the function in the parameter, it would be using the return value of the parameter. Remember functions in JavaScript are first-class citizens, you can use them as parameters, but if you call them, then you will be using their return value. In this case, console.log doesn't actually have a return value, so the parameter in javascript's eyes actually looks like:
function doSomething(text, undefined) {
undefined('Doing something with ' + text);
}
instead of
function doSomething(text, console.log) {
console.log('Doing something with ' + text);
}
jonathanj
UX Design Techdegree Student 9,378 Pointsjonathanj
UX Design Techdegree Student 9,378 PointsThe reason why it's important/necessary to pay attention to the naming conventions when assigning that argument/parament a name other than what was initially used in the getJSON's declaration is b/c...
"Whenever the getJSON function is called, a new scope is created. So the data variable is uniquely defined within each invocation of the onload function handler insuring that it gets executed with the proper data on each iteration of the map." -Guil Hernandez (transcript ~4:23)