Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial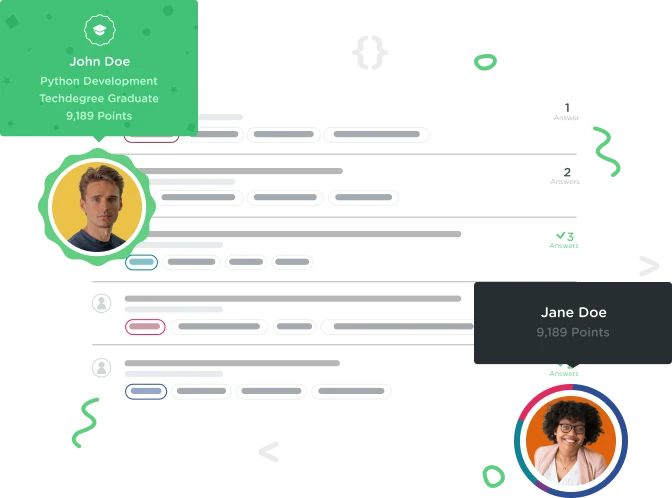
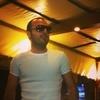
hakan Gülay
8,489 PointsWhy we call load ?
İn this video , we call load like dispenser.load() and then when we write if(!dispenser.isEmpty()){...} it returns true. I couldnt understand how it works .mPexCount was 0 and it means empty right and then when we call dispenser.load() it returns true as well.point which ı didnt understand, does it read mPezCount = MAX_PEZ ? ı confused.
2 Answers
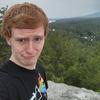
Brendon Butler
4,254 PointsSo you have two different methods here. One is "isEmpty()" which will return a true or false depending on whether or not the dispenser's mPezCount equals 0.
The other method given is "load()" which will set the mPezCount to the maximum capacity that you have set in the variable "MAX_PEZ."
If you wanted to see if the dispenser was empty or not you would check it like this:
if (dispenser.isEmpty()) {
// code to run if the dispenser is empty
} else {
// code to run if the dispenser is full (You don't need this else block, just a demonstration)
}
If you need more information or a better explanation feel free to leave a comment on my post telling me what you don't understand. :)
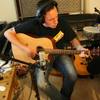
Benjamin Gooch
20,367 PointsHakan,
We don't necessarily have to include the load() method in the Example.java file. As it is written in the lesson, the program starts it's run by first printing "We are making a new Pez Dispenser." using this line:
System.out.println("We are making a new Pez Dispenser.");
Then it moves on to the next line:
PezDispenser dispenser = new PezDispenser("Vault Boy");
which creates our new object (in may case, i'm a huge Fallout fan, so I chose to deviate from the lesson a smidge). Next it displays the name of the character using the value you pass into the PezDispenser() method.
System.out.printf("The dispenser character is %s\n", dispenser.getCharacterName());
Now we get to the part you are asking about.
if(dispenser.isEmpty()) {
System.out.println("It is currently Empty!");
}
It asks whether or not the dispenser is empty and if it is, returns the boolean value of true, which it is, since the default value of 0 for mPezCount is defined when the object is created. It then moves on to the next line and prints the loading text:
System.out.println("Loading...");
followed by running the load() method:
dispenser.load();
and finally it asks again whether or not the dispenser is empty. It is in fact, at this point, full, since we used the load() method. Mine reads like this (again, I took some creative liberties ):
if(!dispenser.isEmpty()) {
System.out.println("It is currently a vessel full of delicious neck-candies.");
}
You don't actually have to write in the load() method so that it is executed on run. You could instead write your code like this:
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are making a new Pez Dispenser.");
PezDispenser dispenser = new PezDispenser("Vault Boy");
System.out.printf("The dispenser character is %s\n",
dispenser.getCharacterName());
if(dispenser.isEmpty()) {
System.out.println("It is currently Empty!");
}
}
}
Notice I left out the "loading..." and calling of the dispenser.load() method. Now, when you run the program, you'll just get this:
We are making a new Pez Dispenser.
The dispenser character is Vault Boy
It is currently Empty!
Now in the console you can type:
dispenser.load();
and it will set mPezCount to 12 because that's what the load() method is programmed to do. I would actually re-include the "Loading..." read out and the re-check in the load() method's block of code in the PezDispenser class so when you execute the method, it says "Loading..." then checks to make sure it functioned properly.
Does that explain your question? I may have been a bit over-thurough, but I wanted to make sure I covered everything. Also, I'm pretty new to Java, so if anyone notices I got something wrong, please let me know!!!
Thanks, Ben
hakan Gülay
8,489 Pointshakan Gülay
8,489 PointsThx for answering :) when ı remove load() function it still works actually ı didnt understand why it is there.it doesnt keep value right ?. in load function, MAX_PEZ gives its value which is 12 to mPezCount ,mPezCount was 0 after that it would be 12. ok but still I still am confused :) am ı right ?
Brendon Butler
4,254 PointsBrendon Butler
4,254 PointsEvery time you compile and run your code, whatever the values started at will remain what they were when you hard coded them in.
If you run the program and change the values, the next time you run the code the values will be what they were before you ran the code the first time. The only time this wouldn't be true, is if you had your program save the values to a file and load them back up the next time you ran the program.
Maybe I'm a little confused as to what you were asking. Sorry :/