Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial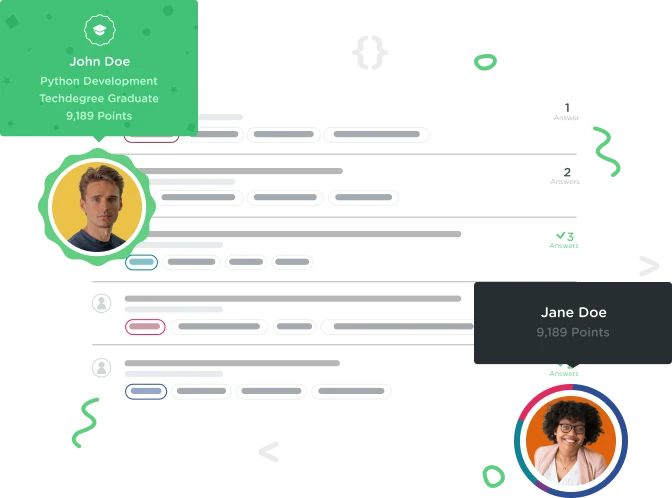
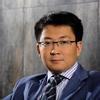
Andrei Li
34,475 PointsWhy we cannot use in for loop just a "letter"? We need "char letter" although we declared the variable in a parameters?
public int getCountOfLetter(char letter) {
for (char letter : tiles.toCharArray()) {
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public int getCountOfLetter(char letter) {
for (char letter : tiles.toCharArray()) {
int count=0;
count++;
}
return count;
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
2 Answers
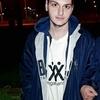
Tornike Shelia
2,781 PointsI might be wrong here and correct me someone if I am , but 'char letter' which is in the parameter and 'char letter' which is in the for loop are different , because it gets created in the for loop and also it gets destroyed after the for loop is finished. So let's say that you have some method and inside that method you have a for loop :
public void someMethod(){
for(int i =0 ; i<5 ; i++){
System.out.println(i); //This is just an example of for loop printing numbers from 0 to 4
}
}
Now from example above you can see that I have created the variable i in for loop and assigned 0 to it. That variable CANNOT be used in other places , it can only be used in the for loop because it's inside of the for loops scope , anything outside that for loops brackets CANNOT see the variable i , so you even can create your own variable outise the for loop and it will be different .
In your example , the for loop creates the variable letter inside the for loops score , and then it assigns that variable to every single word in tiles one-by-one after each iteration .
One more example to understand it better :
public void anotherMethod(){
int[] someArray = {3 ,4 , 5};//just an array of numbers
for(int i : someArray){
System.out.printf(i); //it will print 3 4 5
}//for loop ends here
String i = "This is different variable that has nothing to do with the variable inside the for loop";
System.out.printf(i); //This will print the String i because for loop is ended and we cannot access its variable from for loops outer scope (outside for loop).
}//Method ends here.
To understand it better I suggest you to google - Java scope or Java loops scope , I'm sure you'll find better answers than mine :) Good luck

Daniel Marin
8,021 PointsHi, The purpose of the challenge is different: Here's how you can do it:
public int getCountOfLetter(char letter) {
int count=0;
String tiles = getTiles();
for (char tile : tiles.toCharArray()) {
if(letter == tile) {
count++;
}
}
return count;
}
In your code you are defining the count and setting to 0 every time it runs in the loop also the letter param is used to check if a match is found in tiles.