Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial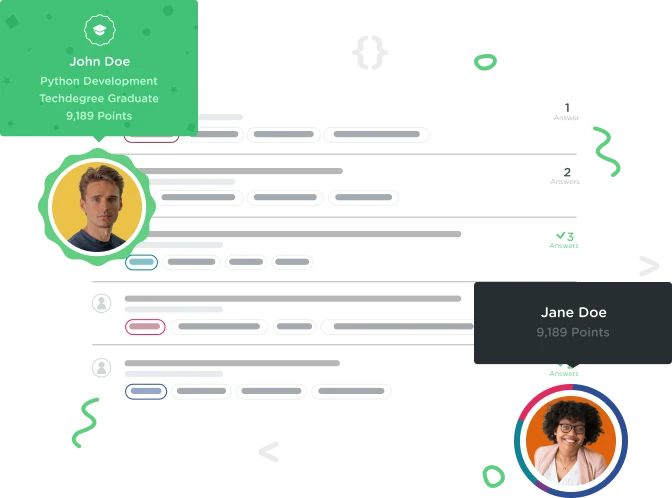

Greg Neborsky
1,020 PointsWhy we need equal operators and zeros in fizz buzz?
I checked Amit's solution and don't get it why he uses equal operators like (i % 3 == 0) or (i % 5 == 0) I mean, if we have a range of 100, then 100 / 5 would be 20, not 0. Would that still be "Buzz" and why? I'm currently trying to make a FizzBuzz generator using Switch statement, but I don't get it yet
8 Answers
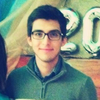
Andres Oliva
7,810 PointsTry this:
for i in 1...30 {
if (i % 3 == 0) && (i % 5 == 0) { println ("FizzBuzz") }
else if (i % 5 == 0) { println ("Buzz") }
else if (i % 3 == 0) { println ("Fizz") }
else { println (i) }
}
Your problem is that when it should be FizzBuzz, it is also Fizz, and your code is entering the fizz if first. If you check FizzBuzz at first it should work as you expect :)
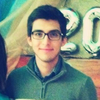
Andres Oliva
7,810 PointsThis "%" is the modulo operator. It gives you the remainder after performing a division.
For example, if you write 23 % 5, the result would be 3, because 23 / 5 = 4 and the remainder is 3.
100 % 5 is 0 because 100 / 5 = 20 and there is no remainder. That means 100 is divisible by 5.

Greg Neborsky
1,020 Pointsthanks a lot, I get it now! the only one thing that I don't understand in this exercise is: I sort of copied Amit's answer, but assistant editor isn't showing me "FizzBuzz" on 15, it shows only "Fizz" instead. Why can it be? Code: for i in 1...30 { if i % 3 == 0 { println ("Fizz") } else if i % 5 == 0 { println ("Buzz") } else if (i % 3 == 0) && (i % 5 == 0) { println ("FizzBuzz") } else { println (i) } }

Daniel Reedy
25,284 PointsThe error is in your control flow. Here is your code formatted.
for i in 1...30 {
if ( i % 3 == 0 ) { // 15 / 3 = 5 with a remainder of 0, this will match and print "Fizz"
println("Fizz")
} else if ( i % 5 == 0 ) {
println("Buzz")
} else if ( i % 3 == 0 && i % 5 == 0 ) {
println("FizzBuzz")
}
You'll need to match for the FizzBuzz cases first.

Greg Neborsky
1,020 Pointslike (15% == 0)?

kerdeseverin
9,688 PointsI just made that comment higher up. I was actually surprised that more people aren't just using %15. Unless Im missing something?

Daniel Reedy
25,284 PointsThe %
modulo operation does not provide quotient but instead provides the remainder.
In your question, 100 / 5
does equal 20
. It divides evenly meaning the remainder is 0
.
Looking at it in pseudo-code:
# Division, checking for quotient
100 / 5 == 20 # True
100 / 5 == 0 # False
# Modulo, checking for remainder
100 % 5 == 20 # False
100 % 5 == 0 # True
If you are curious, check out more on the Division and Modulus in Programming Languages for a really in-depth understanding, or Modulo Operation for something a bit easier to digest.

Greg Neborsky
1,020 Pointsthanks a lot!

Greg Neborsky
1,020 PointsThank you guys so much!

Balkaran Samra
1,341 PointsHaha, I was going to help out too, guess im too late.
Harry Tran
10,618 PointsI wrote it the same way that Amit did, but was wondering if it's just best practices to write the i % 3 && i % 5 as the first check or does that actually affect the answer, like it'll break the code if you do this instead check i % 3 then i % 5 then i % 3 && i % 5
Any thoughts on whether it's just best practice work flow or its actually going to be read differently by the system?
Harry Tran
10,618 PointsOk I just ran a test on my question and it does matter which order you write the conditionals.
Doing if i % 3 == 0 else if i % 5 == 0 else if i % 3 == 0 && i % 5 == 0
completely ignores the final check statement where 15, 30, 45, 60 are not checked against that last condition, so it has to be placed on the top as the first check.

kerdeseverin
9,688 PointsJust a suggestion: If %3 and %5 of the number give zero, you can replace that whole line with %15. It will make the code cleaner and more concise.
for i in 1...30 {
if ( i % 15 == 0 ) {
println("FizzBuzz")
} else if ( i % 3 == 0 ) {
println("Fizz")
} else if ( i % 5 == 0 ) {
println("Buzz")
}

Greg Neborsky
1,020 Pointsthat's neat! Thank's man!
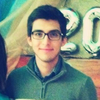
Andres Oliva
7,810 PointsI actually think that writing i % 3 == 0 && i % 5 == 0 is better. Why? Because it makes your intentions clearer to people reading your code. Maybe i % 15 == doesn't make sense to everybody.
One may argue that performance-wise i % 15 == 0 is better, while that's true, it's really nothing that the processor can't handle.

kerdeseverin
9,688 PointsGood point. I do think though that most programmers would be able to understand why the 15 is there easily at a first glance. Yes performance wise it is better, this may be a small chunk of code but the rest of the app may have many other intensive processes so I would always pick performance first to help reduce the load.
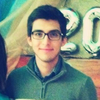
Andres Oliva
7,810 PointsSomething that most computer scientists do in iOS development is over-optimization.
Don't do that! One if is nothing compared to drawing your views on the screen or processing touch input. Really, don't even bother optimizing such simple stuff.
If you have the chance, take a look at the iOS development courses from Stanford in iTunes U. I highly recommend those!

kerdeseverin
9,688 PointsIt depends on the case. If you're working with large enterprise applications, sometimes you have no choice but to optimize. I guess we may have to agree to disagree because using two conditionals doesn't seem needed to me.
Harry Tran
10,618 PointsThanks for pointing that out Kerde, I'm sure it's case by case basis so no right answer here, but I appreciate pointing that out because it's another way to look at the same problem and solving for it with an answer. Thank you to all who commented too. Very helpful for me a newb.