Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial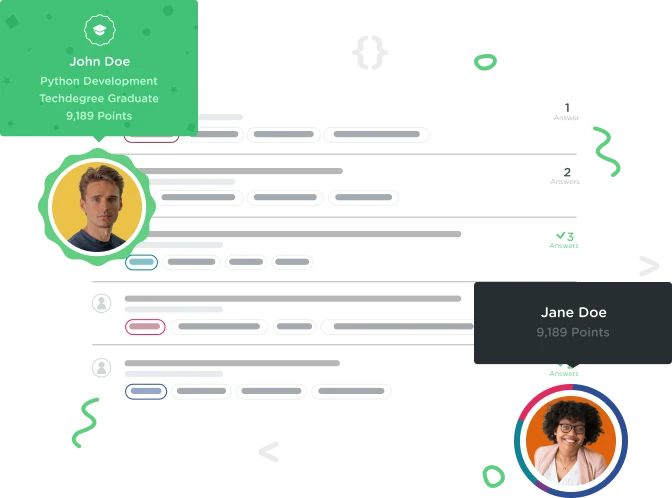
2 Answers
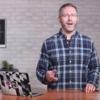
Jay McGavren
Treehouse TeacherYou're referring to the Increment
and Set
methods on the Minutes
type, right?
type Minutes struct {
value int
}
func (m *Minutes) Increment() {
m.value = (m.value + 1) % 60
}
func (m *Minutes) Set(newValue int) {
m.value = newValue % 60
}
If the m
receiver parameter to Increment
were not a pointer, it would receive a copy of the Minutes
value we want to update. Increment
would update the value
field on the copy, and then it would discard the copy.
Instead, Increment
needs to receive a pointer to a Minutes
value. That will allow it to update the field on the original Minutes
value, not the copy.
All of this is the same for the Set
method as well.
This is exactly like our halve
function that needed a pointer parameter in the pointers video, except that in this case we need our receiver parameter to be a pointer.
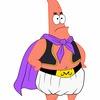
<noob />
17,062 PointsJay McGavren I saw this video again and i understand that in order to update the value and the copy of a value we need to use pointers, because they are not in the same SCOPE? and because of that we add a pointer to Minutes? I would appreciate it if u can give another example :} It is the hardest topic ....
I am a newbie in go, I just finished the course, can u recommend what to do now? which resources u recommend to follow from here? I really want to see more content from treehouse about go as it rapidly growing.. in any case Thanks for taking ur time to answer my question!
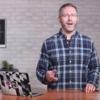
Jay McGavren
Treehouse TeacherThat's right, the variable used as the function parameter or method receiver parameter is not in the same scope as the code that's calling the function/method. So the parameter falls out of scope when the function/method ends, and any changes to it are lost. Unless the function/method takes a pointer parameter, in which case it's modifying the exact same value used in the code that's calling the function/method.
Another explanation of pointers in general is here: https://dave.cheney.net/2017/04/26/understand-go-pointers-in-less-than-800-words-or-your-money-back
Another example with pointer function parameters is here: https://gobyexample.com/pointers
An additional example with pointer method receivers is here: https://tour.golang.org/methods/4
Steven Parker
241,783 PointsSteven Parker
241,783 PointsHappy coding!