Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial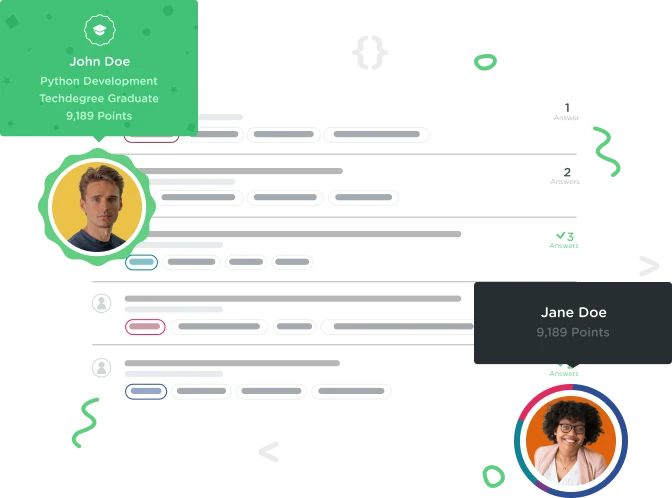

aidanaatrentredi
3,909 PointsWhy when removing setTimeout function inside the Promise, 'value' gets undefined?
Even when we declare it before invoking mathPromise:
const mathPromise = new Promise( (resolve, reject) => {
// resolve promise if 'value' is a number; otherwise, reject it
if (typeof value === 'number') {
resolve(value);
} else {
reject('You must specify a number as the value.')
}
});
const value = 5;
mathPromise
.then(addFive)
.then(double)
.then(finalValue)
.catch( err => console.log(err) )
outputs: 'You must specify a number as the value.'
However, when the value is placed before constructing the Promise, it works as it should:
const value = 5;
const mathPromise = new Promise( (resolve, reject) => {
// resolve promise if 'value' is a number; otherwise, reject it
if (typeof value === 'number') {
resolve(value);
} else {
reject('You must specify a number as the value.')
}
});
mathPromise
.then(addFive)
.then(double)
.then(finalValue)
.catch( err => console.log(err) )
I am a bit confused how it works.
1 Answer
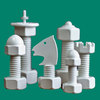
Steven Parker
230,274 PointsWhen you create a Promise, the function passed to it (the "executor") is invoked immediately and asynchronously, but with the understanding that it will at some point call either "resolve" or "reject".
With the timer involved, the code had 1000 ms (tons of time for a computer) to establish "value" before it was tested in the executor. But without it, the test occurs before the variable declaration is reached, so it's still undefined at that point.
Then by defining the variable before creating the Promise, the issue is resolved.
NaiShiuan Zheng
16,881 PointsNaiShiuan Zheng
16,881 PointsI also was confused by this at first, it seems to make people (or me at least) think that the setTimeout is essential inside the promise code, I removed it and had the same "undefined" result as the OP. Moving the const
value = 5;
above the promise like the OP mentioned would be acceptable right? Since this isn't a video, I would suggest making the code examples as simple as possible, other than that it was a great review by Treehouse.edit : actually from the way they made this review (introducing the functions first and then writing out the actual declaration of vars and invoking promises, I kinda get why they did that, so it's not a big issue now, at least people can find answers here)
Steven Parker
230,274 PointsSteven Parker
230,274 PointsEven without the timer, this is a great example of what's called a "race condition", which is always a concern with asynchronous programming.
If you use codepen, you may have seen this joke that also illustrates the issue:
  "Knock, knock."   "Race condition!"   "Who's there?"