Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial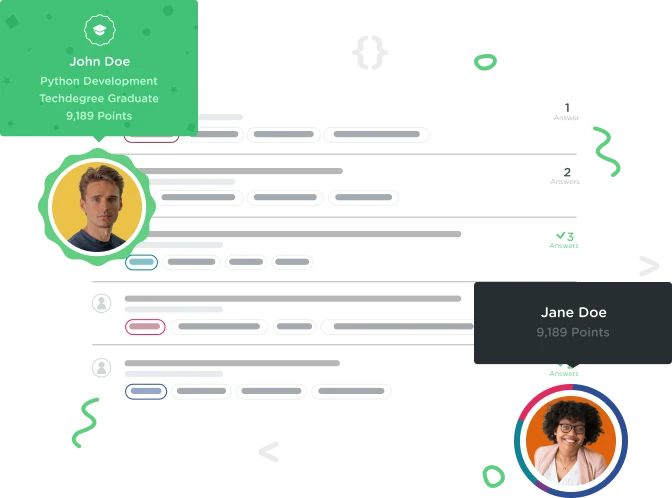

Zachary Davidson
Full Stack JavaScript Techdegree Graduate 14,454 PointsWhy will my reduce method not work without defining the current value at 0
const names = ['Gary', 'Pasan', 'Gabe', 'Treasure', 'Gengis', 'Gladys', 'Tony']; const gNames = names.reduce((sum, name) => { if (name.charAt(0) === 'G') { sum += 1; } return sum; }, 0);
console.log(gNames);
Here is my code. Why does this only work if 0 primes the current value parament at line 7. If I remove 0, the code will not work and will return NaN. I thought current value defaults to 0 but it seems like there is a type issue here?
Just curious, thanks!
1 Answer
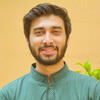
Zaid Khan
12,769 PointsIf you will not provide the initial value, reduce() will execute the callback function starting at index 1, not 0. It's going to skip the first index and move on to other elements of the array.
See the below code:
const names = ['Gary', 'Pasan', 'Gabe', 'Treasure', 'Gengis', 'Gladys', 'Tony'];
const gNames = names.reduce((sum, name) => {
if (name.charAt(0) === 'G')
{
sum += 1;
}
return sum;
});
console.log(gNames)
If you run this in the console, You will get Gary111
.
You see by not giving the initial value of 0 it skipped the first index and go on to perform the condition on the remaining elements. So it Skipped Gary and return all those elements where G matches at index 0 of character which is 3.
From the docs: If no initialValue is supplied, the first element in the array will be used and skipped.
More info at MDN
Also for better readability, you can wrap your code with 3 backticks (```) on the line before and after.
Zachary Davidson
Full Stack JavaScript Techdegree Graduate 14,454 PointsZachary Davidson
Full Stack JavaScript Techdegree Graduate 14,454 PointsGreat answer. Will format my questions with readability in mind in the future as well. Thanks!