Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial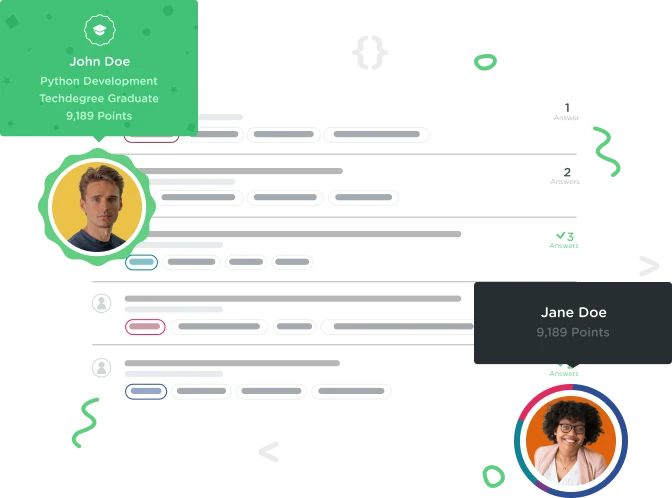

nikmillard
12,703 PointsWhy Won't it Work!?
import random
COLORS = ['red', 'yellow', 'pink', 'green']
class Monster:
min_hit_points = 1
max_hit_points = 1
min_experience = 1
max_experience = 1
weapon = 'sword'
sound = 'roar'
def __init__(self, **kwargs):
self.hit_points = random.randint(self.min_hit_points, self.max_hit_points)
self.experience = random.randint(self.min_experience, self.max_experience)
self.color = random.choice(COLORS)
for key, value in kwargs.items():
setattr(self, key, value)
def battlecry(self):
return self.sound.upper()
This thing if formatting my copied and pasted code from Workspaces all wonky so hopefully you can read it OK.
My problem is I can't get the function that generates the hit_points, experience and color attributes to work or the function to change the sound to upper case. I've typed and retyped the code whilst rewatching the video in Workspaces, Pycharm and the Python 3.5 IDLE and in all cases if I create a monster and call the hit_points, experience or color attributes it tells me it does not exist.
Traceback (most recent call last): File "<input>", line 1, in <module> AttributeError: type object 'Monster' has no attribute 'hit_points'
In Pycharm if you bring up the "show variables" window it shows my monster has a weapon, sound (all in lower case), min_hit_points, max_hit_points, min_experience and max_experience attributes instead of weapon, sound (in upper case) hit_points, experience and color attributes like i'm expecting.
Hopefully someone can point out what's going wrong because I have no clue.
2 Answers

Taylor Schimek
19,318 PointsHey Nik,
From the error you're receiving, it looks like you are instantiating the class incorrectly.
Correct way would be:
my_monster = Monster()
That the error says "type object 'Monster'" makes me think you put:
my_monster = Monster
Don't forget the ()
Hope that helps.

nikmillard
12,703 PointsHi Taylor, Thanks for the help the () makes all the difference, I think I had been looking at the problem for so long I could not see the wood for the trees so to speak, I did not get a chance to get back to this for a coupIe of days so when I got a chance to return I went back a couple of videos in the course and saw what I was doing wrong then came back here and saw your message, the smallest things can break everything which is extremely frustrating when you're a beginner and don't really know what you're doing, I will learn from my mistake which is good.