Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial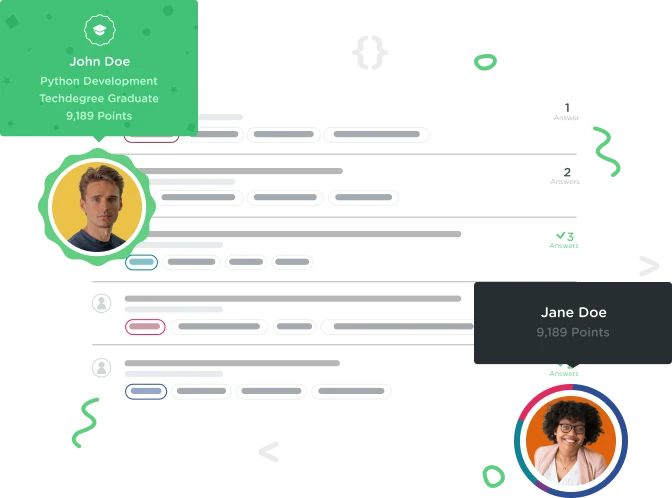
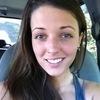
Amber Cyr
19,699 PointsWhy won't my buttons work!
I have tried everything! playButton.onClick(), nextButton.onClick(), and stopButton.onClick() work just fine in the console but my physical buttons do nothing. Iv'e cleared the cache several times because I have had problems in the past related to that. I have gone over the video and code several times and I don't seem to be missing anything but I'm obviously wrong. I'm probably missing something small but I need help finding it. Thanks in advanced!
here's my code:
app.js ''' var playlist = new Playlist();
var hereComesTheSun = new Song("Here Comes The Sun", "The Beatles", "2:54"); var theWall = new Song("The Wall", "Pink Floyd", "4:52"); var wishYouWereHere = new Song ("Wish You Were Here", "Incubus", "3:52");
playlist.add(hereComesTheSun); playlist.add(theWall); playlist.add(wishYouWereHere);
var playlistElement = document.getElementById("playlist");
playlist.renderInElement(playlistElement);
var playButton = document.getElementById("play"); playButton.onClick = function(){ playlist.play(); playlist.renderInElement(playlistElement); }
var nextButton = document.getElementById("next"); nextButton.onClick = function(){ playlist.next(); playlist.renderInElement(playlistElement); }
var stopButton = document.getElementById("stop"); stopButton.onClick = function(){ playlist.stop(); playlist.renderInElement(playlistElement); } '''
playlist.js ''' function Playlist() { this.songs = []; this.nowPlayingIndex = 0; }
Playlist.prototype.add = function(song) { this.songs.push(song); };
Playlist.prototype.play = function() { var currentSong = this.songs[this.nowPlayingIndex]; currentSong.play(); };
Playlist.prototype.stop = function(){ var currentSong = this.songs[this.nowPlayingIndex]; currentSong.stop(); };
Playlist.prototype.next = function() { this.stop(); this.nowPlayingIndex++; if(this.nowPlayingIndex === this.songs.length){ this.nowPlayingIndex = 0; } this.play(); };
Playlist.prototype.renderInElement = function(list) { list.innerHTML = ""; for(var i = 0; i < this.songs.length; i++){ list.innerHTML += this.songs[i].toHTML(); }
}; '''
songs.js ''' function Song(title, artist, duration) { this.title = title; this.artist = artist; this.duration = duration; this.isPlaying = false; }
Song.prototype.play = function() { this.isPlaying = true; }
Song.prototype.stop = function() { this.isPlaying = false; }
Song.prototype.toHTML = function() { var htmlString = '<li '; if(this.isPlaying){ htmlString += "class='current'"; } htmlString += ">"; htmlString += this.title; htmlString += ' - '; htmlString += this.artist; htmlString += '<span class="duration">'; htmlString += this.duration; htmlString += '</span></li>'; return htmlString; } '''
2 Answers
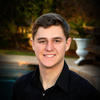
Colton Ehrman
Courses Plus Student 5,859 Pointsonclick is all lowercase like
playButton.onclick = function() {};
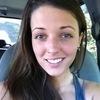
Amber Cyr
19,699 Pointsomg thank you!! i have no idea why the code completion was giving me onClick instead and onClick works in the console but onclick did it!! Thanks again!
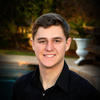
Colton Ehrman
Courses Plus Student 5,859 PointsLol no problem. JavaScript is weird :P Most functions are camel case and then it has some odd function like onclick, I think it has to do with being able to be placed inside html thats why it's not camelcase
Colton Ehrman
Courses Plus Student 5,859 PointsColton Ehrman
Courses Plus Student 5,859 Pointsplaylist.js
song.js