Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial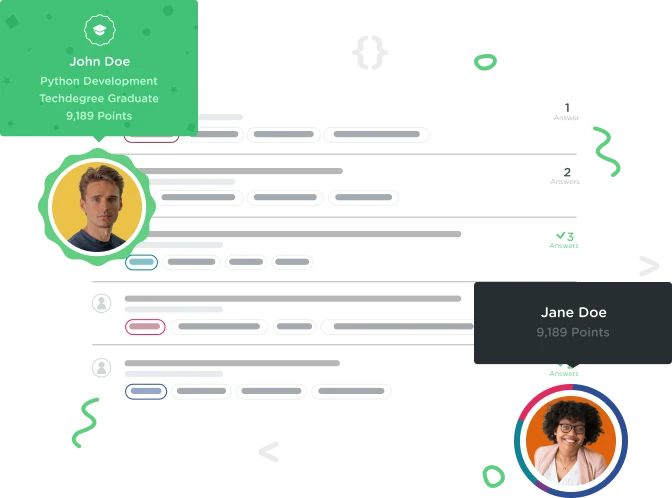
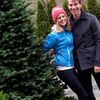
Jennifer Hughes
11,421 PointsWhy won't my list items appear correctly?
Although my array contains three items, only one appears on the screen in my printed list.
Any ideas?
var correctAnswers = 0;
var incorrectAnswers = 0;
var correctQuestions = [];
var incorrectQuestions = [];
function print(message) {
document.getElementById('numberOfCorrectAnswers');
numberOfCorrectAnswers.innerHTML = message;
}
function buildList(array) {
var listHTML = '<ol>';
for (var i = 0; i < array.length; i++) {
listHTML = '<li>' + array[i] + '</li>';
}
listHTML += '</ol>';
return listHTML;
}
questions = [
['How many states are in the United States?', 50],
['What is the oldest state?', 'delaware'],
['Who was the first presdient?', 'george washington']
]
for (var i = 0; i < questions.length; i++) {
answer = prompt(questions[i][0]);
if(answer.toString() === questions[i][1].toString()) {
correctAnswers++;
correctQuestions.push(questions[i][0]);
}
else {
incorrectAnswers++;
incorrectQuestions.push(questions[i][0]);
}
}
console.log(correctQuestions);
message = 'You got ' + correctAnswers + ' question(s) right';
message += '<h2> You got these questions correct:</h2>';
message += buildList(correctQuestions);
message += '<h2> You got these questions incorrect:</h2>';
message += buildList(incorrectQuestions);
print(message);
2 Answers
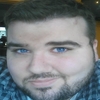
Marcus Parsons
15,719 PointsHey Jennifer,
In your print
function, you have to either set a variable equal to the document.getElementById()
statement or just combine the two:
function print(message) {
var numberOfCorrectAnswers = document.getElementById('numberOfCorrectAnswers');
numberOfCorrectAnswers.innerHTML += message;
}
Or
function print(message) {
document.getElementById('numberOfCorrectAnswers').innerHTML += message;
}
Edit: modified to reflect changes below. Added += to function.
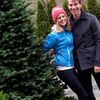
Jennifer Hughes
11,421 PointsHi Marcus,
Thanks for your help. Two things:
I put toString() because the answers could come in as numbers. When I leave toString() off, it does not register that 50 is the correct answer to the first question.
Secondly, it still is not working properly. If I answer all three questions correctly, it only prints one of the questions to the screen, the last question.
Any additional thoughts?
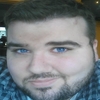
Marcus Parsons
15,719 PointsYou need to have += in your print function. I didn't even think about that at first. I just knew the code would work lol. You don't have to put to string on your answer variable, however, because a prompt will always come in as a string. I would instead encapsulate the number 50 as a string, instead of calling toString(). It's much faster that way because you're not having to call methods to compare the data.
function print(message) {
document.getElementById('numberOfCorrectAnswers').innerHTML += message;
}
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsOh, also you don't need to put the
toString()
method on your answer or questions since they're already strings.