Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial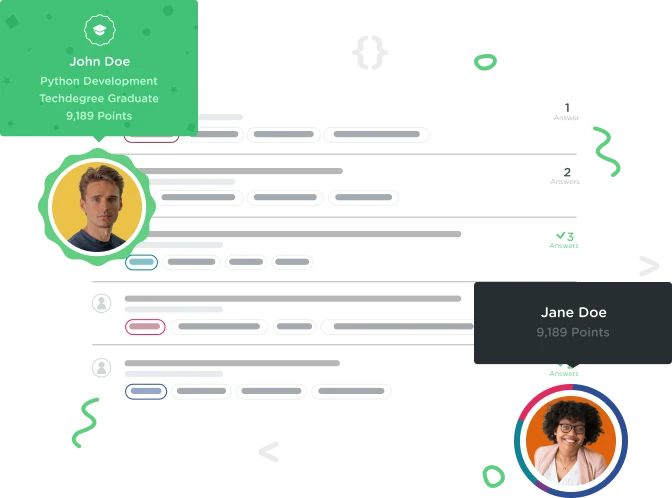
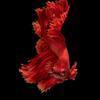
Michael Williams
Courses Plus Student 8,059 PointsWhy won't .querySelectorAll work in this challenge?
I know the right answer:
if (e.target.tagName == "INPUT") {...}
However, why won't .querySelectorAll
work in this situation? I thought it was selecting the right elements, but maybe my syntax is wrong?
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<section>
<h1>Making a Webpage Interactive</h1>
<p>JavaScript is an exciting language that you can use to power web servers, create desktop programs, and even control robots. But JavaScript got its start in the browser way back in 1995.</p>
<hr>
<p>Things to Learn</p>
<ul>
<li>Item One: <input type="text"></li>
<li>Item Two: <input type="text"></li>
<li>Item Three: <input type="text"></li>
<li>Item Four: <input type="text"></li>
</ul>
<button>Save</button>
</section>
<script src="app.js"></script>
</body>
</html>
let section = document.getElementsByTagName('section')[0];
section.addEventListener('click', (e) => {
if (e.target.querySelectorAll == "[TYPE=TEXT]") {
e.target.style.backgroundColor = 'rgb(255, 255, 0)';
}
});
2 Answers
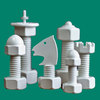
Steven Parker
231,268 PointsThere is a method used to select elements named "querySelectorAll", but it's not the name of a property. Also, in this case you already have the element as "e.target". So you don't need to select anything, you just need to test the element you have.
If you wanted to perform this task by testing the element type instead of the tag name, you could do it this way:
if (e.target.type == "text") {
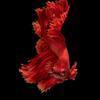
Michael Williams
Courses Plus Student 8,059 PointsSteven Parker, thank you for all your help. I've rewatched the Event Object video, but one thing I'm still not understanding is what is it that's being passed through as an argument in the "e" parameter? Normally, the parameter would already be defined as a function, variable, etc. and we would type it's name when passing through. But we're doing neither of those things in this instance.
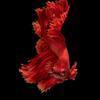
Michael Williams
Courses Plus Student 8,059 PointsSteven Parker I thought about it one more second.... and in the code example above, is it the section
variable that's being passed in the e
parameter?
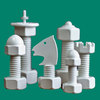
Steven Parker
231,268 PointsThe parameter passed to an event handler ("e" here) is always an Event object. It has a target property which contains a reference to the element that dispatched the event. Since this is a "click" handler, it would be the thing that got clicked on. We don't know in advance what that might be β it could be any element within the section. It's not likely to be the section itself, since a section doesn't render anything visible on the screen to click on.
Michael Williams
Courses Plus Student 8,059 PointsMichael Williams
Courses Plus Student 8,059 PointsFirst off, that's where I went wrong, confusing the method with a property. So that makes sense. However, I have several more questions:
(e)
be anything I want it to be? In the videos Gil used(event)
. So I thought it always had to be event until this example.e
parameter? Normally, with a function, we'd pass something different in through there.e.target
automatically know which element I'm pointing it to independent of the if statement? Because I don't see anything telling it where the element is outside of that condition?Steven Parker
231,268 PointsSteven Parker
231,268 Pointstarget
property of an event object is a reference to the object that dispatched the event.And for fun and extra practice give Advent of Code a try.
Happy holidays!