Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial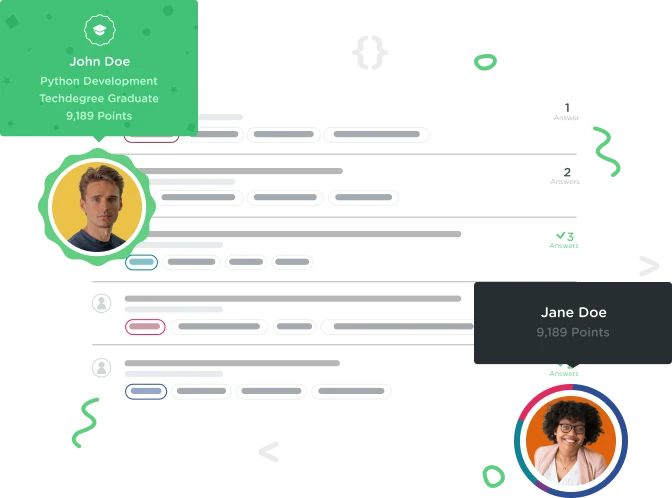
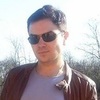
catalinsebastian
9,195 Pointswhy won't switch work?
i tried a similar approach using a switch statement which apparently won't let me use the "==" causing my playground to give me an error because binary operator cannot be applied to operands of type Bool and Int
my approach would look something like this
for number in 1...100 {
switch number {
case (number % 3 == 0 ):
println("Fizz")
case (number % 5 == 0 ):
println("Buzz")
case (number % 3 == 0 ) && (number % 5 == 0 ):
println("Fizz Buzz")
default:
println(number)
}
}
3 Answers
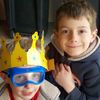
Chris Stromberg
Courses Plus Student 13,389 PointsFrom the IOS developers library: "An if statement is used for executing code based on the evaluation of one or more conditions".
if a == b {
Enter your code here that you want to do.
}
The code above does two things.
It evaluates your condition, "does a == b?"
If this evaluation is true, it does whatever is in the code block.
With a switch statement it is comparing values of the same type and looks for a match.
The code below we are comparing the constant "letterA" to each case statement. If we have a match, the code that follows is executed.
let a = "a"
let letterA = a
switch letterA {
case "a" : println("This is an a")
case "b" : println("This is a b")
case "c" : println("This is a c")
default : println("I don't know what letter this is")
// "This is an a"
// This is printed because letterA matches "a"
//If we substituted your code.
for number in 1...100 {
switch number {
case (number % 3 == 0 ):
println("Fizz")
//This would be asking if we have a number does it match true or false? This
// doesn't compute because you are trying to match a Int type with a
//boolean type.
In summary:
The "if" statement evaluates a conditon and if the condition is true it executes the code within its block.
The "switch" statement matches types, and if it finds a match, it executes the code that follows.

Jack Hayton
2,012 PointsNo, this is not what Amit's code is saying. The if statements work as follows:
x represents the number
Is the following condition true?: (x) % 3 = 0 AND (x) % 5 = 0 If yes, then print FizzBuzz
Is the following condition true?: (x) % 3 = 0 If yes, then print Fizz
Is the following condition true?: (x) % 5 = 0 If yes, then print Buzz
So the difference of what is happening to what you had previously stated is that the condition checks whether or not it is met. If the condition is true, then the code is executed, if the condition is false, nothing is executed.
Hope this helps.
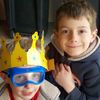
Chris Stromberg
Courses Plus Student 13,389 PointsFrom the IOS developer library, "In its simplest form, a switch statement compares a value against one or more values of the same type".
The line below will do whatever is in the block 100 times. I know you know that already!
for number in 1...100 {
}
Within that block you are running the switch statement 100 times. However what you are asking it to do does not make sense. Look at the code below.
switch number {
case (number % 3 == 0 ):
println("Fizz")
You are asking it to look a number and compare it too a boolean. For example:
If (0) is equal to False print "Fizz".
If (1) is equal to False print "Fizz".
If (2) is equal to False print "Fizz".
if (6) is equal to True print "Fizz".
You can't evaluate that, they are of different types.
catalinsebastian
9,195 Pointscatalinsebastian
9,195 Pointsthat cleared it up for me ,
what confused me was Amit saying during the video that switch statements with their versatility are "favoured" over if statements so it felt like comparing them (being alike) , i thought they do work roughly the same way , and using switch would be a more effective way to write that code, i understand the difference now.
thank you very much, i appreciate the help !