Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial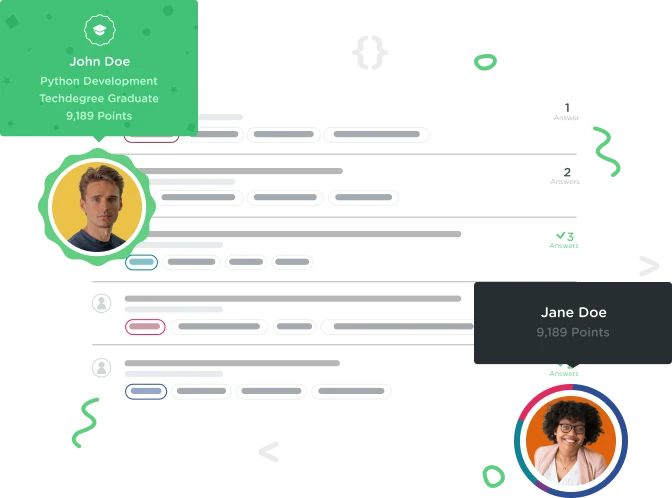

Samar Khanna
1,757 PointsWhy won't switching changing the initial value of "found" to "false" work?
this the code:
func searchNames (#name:String) -> (Bool,String) {
let myFriends = ["h","i","j","k","l"]
var found = (true, "\(name) is a friend of mine")
for n in myFriends {
if name != n {
found = (false,"\(name) is not a friend of mine")
}
}
return found
}
searchNames(name: "i")
The result pane is showing me false
2 Answers
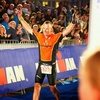
Steve Hunter
57,712 PointsHere's how I would achieve the same aim as your code:
func mySearchNames (#name: String) -> (Bool, String) {
let myFriends = ["h","i","j","k","l"]
for n in myFriends {
if n == name {
return (true, "\(name) is a friend of mine")
}
}
return (false, "\(name) is not a friend of mine")
}
Here, we just go straight into the loop without declaring a variable to hold the two possible results. We have one test, we look if the current element of the myFriends
array is the same as the name
parameter passed into the function.
If the test is true
, i.e. n == name
then we immediately return the tuple you required. That leaves the loop immediately.
If we get to the end of the loop without running the return
line, that means that none of the n
elements equalled name
. So, we return the opposite tuple and exit the method.
I hope that makes sense. This method has fewer lines of code and a clear line of execution. The logic of the tests doesn't require double negative results to occur so the execution route is clearer and uses simpler logic.
Steve.

Samar Khanna
1,757 PointsThank you so so much. You have been really helpful. I didn't understand your first 2 answers, but this one makes sense
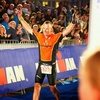
Steve Hunter
57,712 PointsBecause the loop iterates through the whole array of letters. The last one in the array is l
. That doesn't equal i
so it returns, correctly, false
.
Also, there's nothing to set the resut back to true
. So, before the first iteration has happened, the start of your found
variable is true
. The first iteration then occurs. In that scenario, name != n
so found
is set to false
.
The next iteration name == n
but that just skips the if
block, rather than doing anything to the string/tuple so found
remains the same to the end of the for loop, and false
is returned.
You need to fix two things. First, you need to have the test set both conditions. Second, you need to get out of the loop as soon as the desired result is reached, rather than testing the whole array. i think you may need to reverse the test too, rather than looking for inequality, look for equality.
I'll give you a couple of fixes. One better than the other.
Steve.
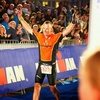
Steve Hunter
57,712 PointsUsing your code as a basis, although I stand by the above, adding a couple of lines gets the result you are expecting:
func searchNames (#name:String) -> (Bool,String) {
let myFriends = ["h","i","j","k","l"]
var found = (true, "\(name) is a friend of mine")
for n in myFriends {
if name != n {
found = (false,"\(name) is not a friend of mine")
} else {
found = (true, "\(name) is a friend of mine") // set the tuple again
return found // get out of the loop before the next test is true, i.e. name != n
}
}
return found
}
searchNames(name: "i")
This now exits the loop as soon as name != n
is false
and returns true
.
That double/triple negative is one of the problems with this code. If you are looking to return true
when a !=
test returns a false
the code is confused and some simpler syntax is likely to create much clearer code. Currently you are testing for when your not equal condition is false so you can return true.
Steve.
Samar Khanna
1,757 PointsSamar Khanna
1,757 PointsSorry the code looks a bit odd, but it starts from "func searchNames..."