Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial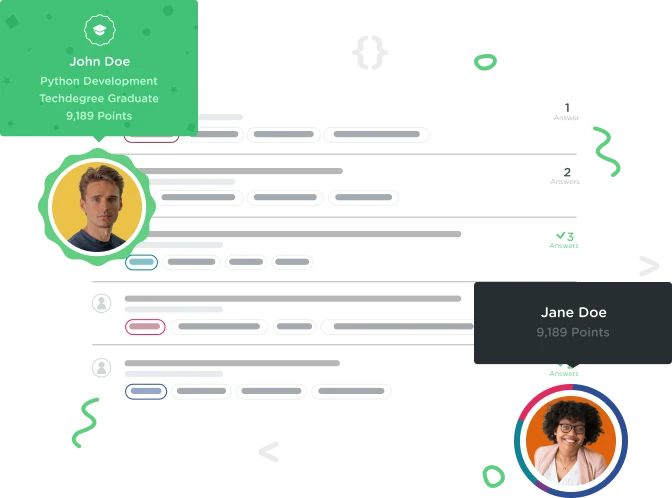

Akhil Matabudul
2,386 PointsWhy won't the second part work????
So, I managed to correct the first part of the question but unfortunately I can't seem to get the second part to work. And I can't seem to figure out why. Could anyone shed some light?
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
import com.example.BlogPost;
public class TypeCastChecker {
/***************
I have provided 2 hints for this challenge.
Change `false` to `true` in one line below, then click the "Check work" button to see the hint.
NOTE: You must set all the hints to false to complete the exercise.
****************/
public static boolean HINT_1_ENABLED = false;
public static boolean HINT_2_ENABLED = false;
public static String getTitleFromObject(Object obj) {
// Fix this result variable to be the correct string.
if((String)obj instanceof String)
{
String result = "";
}
return (String)obj;
if((BlogPost)obj instanceof BlogPost)
{
return (BlogPost)obj.getTitle();
}
return (BlogPost)obj.getTitle();
}
}
1 Answer

Collin Halliday
Full Stack JavaScript Techdegree Student 17,491 PointsYou probably figured it out by now, but I thought I'd respond anyway so your question doesn't have 0 answers.
First, you can declare and initialize your result variable (i.e. String result = "";) at the very top of getTitleFromObject(). Then, within the respective scopes of your two if statements, set the proper value of the result variable. You have already type cast obj to String appropriately in your first return statement, but you don't want that cast to happen unless the condition in the first if statement is true. In other words, you do not want your method to try to cast obj to a String when obj is not a String. Because your return statement is placed outside of the code block for your first if statement, it will run regardless of whether or not the condition in your first if statement is true. As such, in the code block of your first if statement, set the result variable to the cast you coded above for your first return statement, then return the result variable at the very bottom of the method. That way, you give your code a chance to evaluate both conditional expressions in your two if statements before returning.
Second, you don't want to type cast within the conditional expressions in your if statements. You want to leave that for your return statements (i.e. if(obj instanceof BlogPost)). In fact, this exercise probably should not allow students to move forward with a type cast in the if statement conditional expression, because it makes your code vulnerable to exceptions. For instance, apart from the issues discussed below, your code above will run as long as you pass a String as the argument to getTitleFromObject(). However, if you pass another object, such as a BlogPost, as the argument, you will get a ClassCastException, because a BlogPost object cannot be cast to a String. The same problem applies to your second if statement. Assuming you were to make the changes I suggest herein but keep the cast to BlogPost in the conditional expression of your second if statement, your code will run as long as you pass a String, a BlogPost object, or an object that extends BlogPost as the argument to getTitleFromObject(). If you pass in a different object, you will again get a ClassCastException, because BlogPost cannot be cast to a completely different object. Even if the code runs when you pass a BlogPost object to getTitleFromObject(), including the cast in the if statement expression is a little redundant.
Finally, your cast to BlogPost in the second return statement is very close, but it needs a little work. I struggled with this part the first time I did this exercise too. If passed a BlogPost object, getTitleFromObject() should return the value of the BlogPost object's title variable. Because BlogPost's title variable is private, you must use its getter method to access title. There are two ways to call a method from outside of its class. If the method is static, you can call it through its class name using dot notation (i.e. BlogPost.getTitle()). Because getTitle() is not static, you need to use an object reference to call it from within getTitleFromObject(). Your code above attempts to call getTitle() on obj and cast obj to BlogPost, but obj does not have a getTitle() method, BlogPost does. As such, you want a BlogPost object reference from which to call its getTitle method. To get this reference, inside the code block of your second if statement, declare a BlogPost object reference and set it equal to the cast from obj to BlogPost (i.e. BlogPost blgPst = (BlogPost)obj;). Then, call getTitle() on the BlogPost object reference and set it equal to the result variable (i.e. result = blgPst.getTitle()).
Your return statement at the very end of getTitleFromObject() should then return the value of the result variable regardless of whether or not a cast took place and regardless of which cast took place.
I know that's a lot of info. I hope it's at least somewhat helpful. Best of luck!