Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial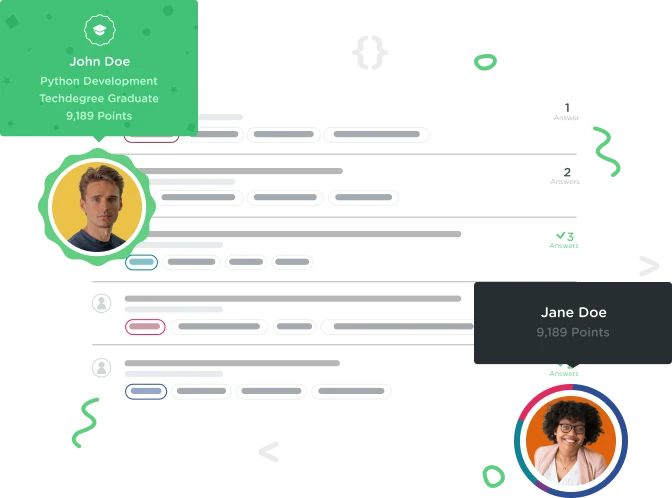
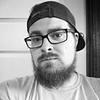
Andrew Minert
3,984 PointsWhy wont this code work?
Need help with finding out why this won't compile.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int tums = 0;
string nums = Console.Read();
int times = Int32.Parse(nums);
int total = int tums + int times;
while (total >= 1 )
{
Console.Write("yay");
total = total - 1;
}
}
}
}
2 Answers
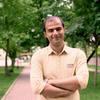
S.Amir Nahravan
4,008 PointsYou can change your code like it
Console.Write("Enter the number of times to print \"Yay!\": "); string nums = Console.Read(); int times = (int)nums;
while (times>0 )
{
Console.Write("yay");
times--;
}

Michel Näslund
18,583 PointsThis compiles for me.
I just changed Console.Read() to Console.ReadLine() and Console.Write() to Console.WriteLine(). Console.WriteLine() will write every "yay" on a new line while Console.Write() prints like yayyayyay...
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
int tums = 0;
string nums = Console.ReadLine();
int times = Int32.Parse(nums);
int total = tums + times;
while (total >= 1 )
{
Console.WriteLine("yay");
total = total - 1; // you could write total--; or total -= 1;
}
}
}
}
Andrew Minert
3,984 PointsAndrew Minert
3,984 PointsThat is absolutely a cleaner way to write it. When I run the code, regardless of the number I type in, it still only prints "yay" once.
S.Amir Nahravan
4,008 PointsS.Amir Nahravan
4,008 Pointsyou don't need to convert to int again and this statement is not correct like it :
int total = int tums + int times; // Incorect statement
Correct is:
int total = tums + times;
Or
int total = (int)tums + (int)times;
Andrew Minert
3,984 PointsAndrew Minert
3,984 PointsPerfect, I corrected that as well, thank you. The same problem is still persisting. Any suggestion on how to make it repeat the word "yay" for the amount that the user types in?