Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial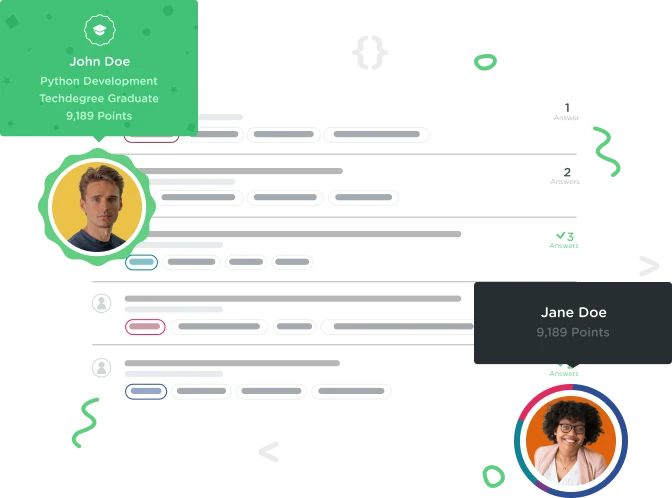
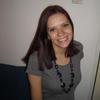
Ivana Lescesen
19,442 PointsWhy won't this work? Thank you for your help :)
Dear Jennifer Nordell would you please be so kind to review my code. Thank x100000 :)
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
var search;
var message = '';
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
while (true) {
search = prompt("Search for a student. Type a name of a student or 'quit' to exit");
search = search.toLowerCase();
if ( search === 'quit') {
break;
} else if ( students.name === search) {
message = studentListing (data);
print(message);
}
else {
print( search + ' is not a student');
}
}
function studentListing (data) {
for (var i = 0; i < students.length; i+= 1) {
var html = " ";
html += "<h2>Student: " + students[i].name + "</h2>";
html += "<h3>Track: " + students[i].track + "</h3>";
html += "<h3>Achievements: " + students[i].achievements + "</h3>";
html += "<h3>Points: " + students[i].points + "</h3>";
return html; }
}
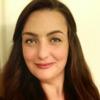
Jennifer Nordell
Treehouse TeacherJohn Erickson Hi there! First, I didn't post the question. And while I agree it's a little odd that in one place, the numbers are integers and in the others they're strings, in the end it makes absolutely no difference because we're not using the points and badge numbers to do any mathematical operations.
4 Answers
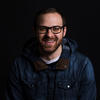
Josh Cummings
16,310 PointsHi Ivana,
If you take a look at your while loop, you have an if statement that checks for students.name
where students is an array of objects. If you run console.log(students.name);
you will get undefined because students
is an array, not an object. If you instead run console.log(students[0]);
you will get a student object at index 0 of the array. Therefore, running console.log(students[0].name);
will give you the string "Dave".
This means that instead of an if/else statement, you would need to loop through the array of objects to find a match.
I have refactored your code with comments to give you an example.
var students = [{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
}, {
name: 'Jody',
track: 'iOS Development with Swift',
achievements: 175,
points: 16375
}, {
name: 'Jordan',
track: 'PHP Development',
achievements: 55,
points: 2025
}, {
name: 'John',
track: 'Learn WordPress',
achievements: 40,
points: 1950
}, {
name: 'Trish',
track: 'Rails Development',
achievements: 5,
points: 350
}];
var search;
var message = '';
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
while (true) {
search = prompt("Search for a student. Type a name of a student or 'quit' to exit");
search = search.toLowerCase();
if (search === 'quit') {
break;
}
// edited
var studentFound = false; // flag to see if a student was found
for (var i = 0; i < students.length; i += 1) {
var student = students[i]; // the current student in the loop
var studentName = student.name.toLowerCase(); // get the name as lowercase
if (studentName === search) {
message = studentListing(student); // pass in the current student
print(message);
studentFound = true; // change our flag
}
}
if (!studentFound) { // if no students were found
print(search + ' is not a student');
}
// end edits
}
// changed this
function studentListing(student) { // we need to pass in a student object
var html = "";
html += "<h2>Student: " + student.name + "</h2>"; // in our loop, this will be the current student's name
html += "<h3>Track: " + student.track + "</h3>";
html += "<h3>Achievements: " + student.achievements + "</h3>";
html += "<h3>Points: " + student.points + "</h3>";
return html;
}
Hope this helps!
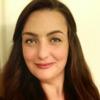
Jennifer Nordell
Treehouse TeacherAh, I see Josh Cummings got to it before I did! Good job!
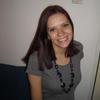
Ivana Lescesen
19,442 PointsJennifer Nordell Aww you are going to make me cry. That is so nice of you. Thank you so much, you just made my day. :) I was really starting to doubt myself, so it feels really good hear positive feedback from someone :) You're the best :)
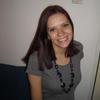
Ivana Lescesen
19,442 PointsThank you Josh Cummings and Jennifer and John Erickson. So you are saying that we do it the why i did because it is an array and not an object. And even if we had called it in a correct manner it would not work because it would only display one name e.g. Dave, but we need it go through all the student names and that is why we have to use a for loop?
You are saying the while loop can only provide one student at a time and does not check the whole list.
Correct me if I am wrong :)
Thank you all for you time, you are truly amazing :)
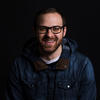
Josh Cummings
16,310 PointsThat's correct.
Items in an array can be accessed by index like so: arrayName[i]
Properties in an object can be accessed with dot notation like so: objectName.property
The while loop here, however, is just there to keep the prompt going until you type "quit". That's why it's
while (true)
While loops are used pretty sparingly in JavaScript in my experience, but here is another example of a while loop:
var i = 1;
while (i <= 3) {
console.log(i);
i++;
}
// output:
// 1
// 2
// 3
We used a for loop so we could iterate through the array and find all the objects to see if there was a name that matched our search.
For loop example:
var numbers = [1, 2, 3]
for (var i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
// output:
// 1
// 2
// 3
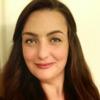
Jennifer Nordell
Treehouse TeacherWell, I know what Josh meant, but technically speaking, an array is an object in JavaScript. Here is some documentation: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array
But what you have here, is an array of objects. Each student is an object but to access a student you have to give the position it resides in the array. And that's why he used the for loop. It starts at 0 and accesses that student array all the way down to the end. But student.name would not produce a result (as described by Josh) because there is no key "name".
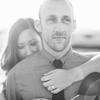
John Erickson
Full Stack JavaScript Techdegree Student 3,916 PointsNo need to thank me, I didn't do much. I'm trying to look into other problems to see if I can take the little that I know and help. It is nice however to read others comments and have some idea as of what they're talking about.
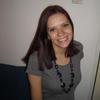
Ivana Lescesen
19,442 PointsThank you guys so much for answering my questions and for your support :)
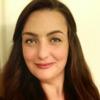
Jennifer Nordell
Treehouse TeacherIt's a pleasure! And let me tell you the truly great thing about your questions, Ivana Lescesen. They tend to be these big idea/concept questions. That's the truly importantly part. The syntax, and where to put a semicolon etc will become second nature after a while. But it's the concepts that elude many people. Hang in there! You're doing fantastic!
John Erickson
Full Stack JavaScript Techdegree Student 3,916 PointsJohn Erickson
Full Stack JavaScript Techdegree Student 3,916 PointsHey Jennifer,
So I'm new to code, so I'm certain I'm wrong, however would it have anything to do with you making achievements and points strings for every student outside your first, that being Dave. I would assume you would want those to be numbers rather than strings, right? Regardless, that could be causing a issue.