Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial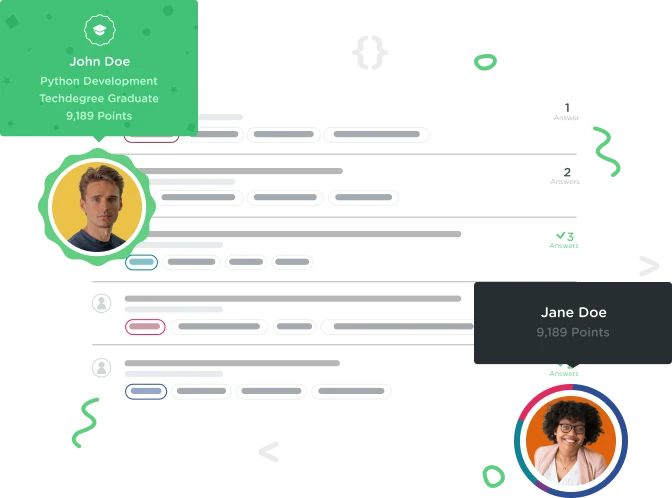

keith frazier
Python Development Techdegree Student 5,771 PointsWhy wouldn't I use this as real code? [4:30]
Around 4:30, Kenneth mentions that the NumString class he creates wouldn't be preferable as actual code. Now, excuse me for taking a beginners python course and not being able to make all of the conclusions Kenneth, a versed developer, makes in all of his videos. I need a little more clarification as to why this isn't pythonic in a real world application. Any help is greatly appreciated!
P.S. Every video should assume you only the know the knowledge obtained in the videos prior. I don't know why Kenneth assumes the student knows functions, modules, and other things that have never been taught in the sequence of Python Techdegree course material. Am I missing something? It seems like all of his videos were filmed separately from the curriculum prior, and shoved into the python course with a "It'll do" attitude. I, like many others, am trying not to be discouraged by this disarray.
eodell
26,386 Pointseodell
26,386 PointsI understand the confusion here. The "magic"/"dunder"/"double underscore" methods that Kenneth is demonstrating is Python's version of a very important OOP concept (method overloading). Essentially, this allows you to change the default behavior of your class in relation to various uses.
Take the following Person class:
This script would produce the following error:
TypeError: object of type 'Person' has no len()
this is because the Person class is not an iterable and the len behavior hasn't been defined.
So if we change our class to something like:
We would get the expected output:
This is because we specified to always return the integer 2 when using the len property. This is probably not useful and wouldn't likely be implmented if you were to use this Person class. What kenneth did was override many common double underscore methods to return
when the int or float methods were called. What if the person implementing the class provided the string "Hello" as the init value when instantiating the class? This can result in unhandled ValueErrors. In a real production application, it is best to only overload methods when you have a specific need or purpose and have properly handled any errors. Of course, Kenneth was just doing a demo so no harm, no foul.
The concept of method overloading is a very important concept of OOP and is would be well worth your time to look into it and spend time reading documentation or watching videos. It can be overwhelming and you may not get a full grasp at first. If you keep using OOP, you'll find that you will eventually need to use these methods and then it will help you understand how and why to use them.
I hope this helps.