Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial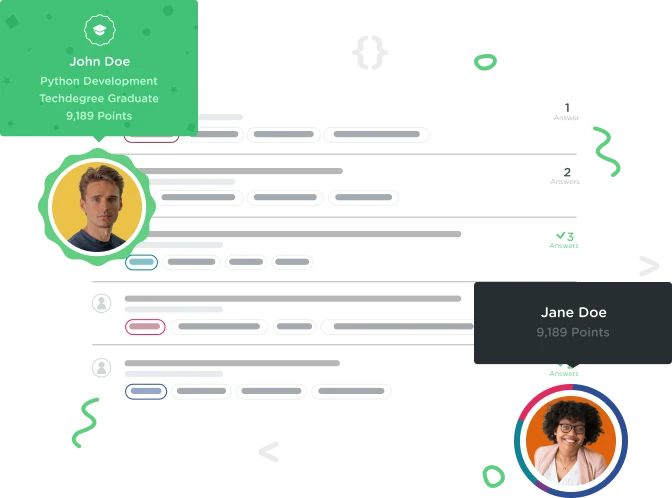

Kyle Salisbury
Full Stack JavaScript Techdegree Student 16,363 PointsWhy write datetime twice?
can someone explain to me why we write "datetime" twice in "datetime.datetime.now". I know that it gives us the time but why can we not just say datetime.now?
2 Answers

Jon Mirow
9,864 PointsHi there!
It's because you're importing the datetime module, a file like file.py, and inside that module you're selecting the class called datetime, from there you're selecting the now() method. So it goes:
- datetime - tells python to select in the datetime module
- datetime - tells python to select the class datatime in that module
- now - tells python to select the method now in that class
- () - execute that module.
For example, here's a file named... bob.py:
class Bob:
_name = Bob
@classmethod
def bob(cls):
cls()
return cls._name
It's pretty basic I know, but if I wanted to use this in my python file i would do:
import bob
name = bob.Bob.bob()
You're right it looks a bit silly. That's why often people do things like:
from datetime import datetime as dt
time_now = dt.now()
or
import datetime as dt
time_now = dt.datetime.now
Basically all you have to remember is that in python the dot means we're going one level inside the object to the left of the dot. Again, for example:
print(object.__class__.__name__)
Here from left to right we select the main object class (where all classes come from), then inside that class there's a __class
__ method which is actually a refrence to the type of the class, so we can step inside that again and get to __name
__ which will return the name of the type.
Hope it helps :)
PS if you're interested you can see the actual datetimemodule file used by cpython here: https://github.com/python/cpython/blob/master/Modules/_datetimemodule.c It's written in C not python, but the principle is the same :)

Kyle Salisbury
Full Stack JavaScript Techdegree Student 16,363 PointsThanks Jon! Great explanation!