Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial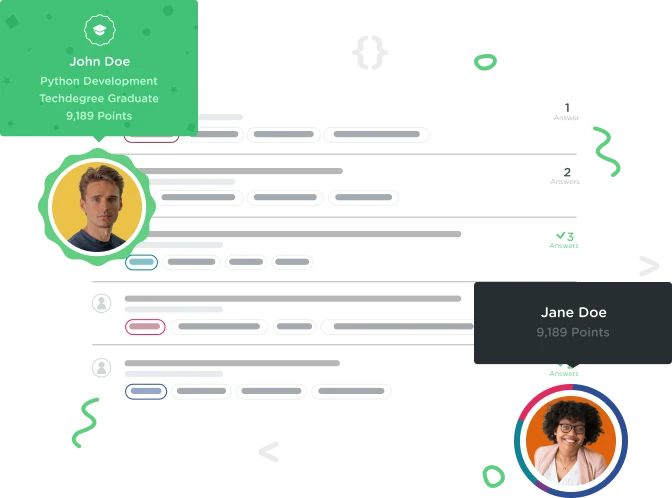
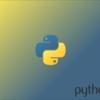
Kevin Faust
15,353 Pointswhyd we put throws IOException after the method???
we never did this before so why did we put a throw IOException after the method? and then whyd we put a try catch block later on? in what way would the promptAction() method produce an error? we are simply just taking an input and storing it as choice so im not sure where an error can occur. help on this would be appreciated
3 Answers
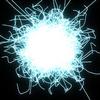
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Kevin,
You're correct on this. Some methods automatically throw an exception, especially buffered reader classes. You want to surround everything in a try catch block so that you have a chance to correct this and be caught by your program before the exception crashes and quits the program.
public int parseInput throws IOException { // define a method that throws your exception
int number;
BufferedReader reader = new BufferedReader(new inputStreamReader(system.in));
String numberChosen; // create your variables
System.out.println("Please enter a number");
try {
numberChosen = reader.readLine(); // read the input put into the program
number = Integer.parseInt(numberChosen); // try to change what was given into an integer.
System.out.printf("Your number is %d", number);
return number; // if successful return the interger passed in
} catch {
// if an integer was not able to be parsed, the catch block is thrown reminding the user to enter a number
System.out.println("Sorry you must enter a number.");
}
}
To move forward on the example above, let's say the user inputted a "a" they would get the catch block that tells them that they must through a number. However if they entered a 3 then they would never see the catch block and the try block would complete successfully and the value of the number that they inputted would be returned by the method.
Thanks hope this helps, let me know if it doesn't.
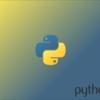
Kevin Faust
15,353 Points*update
so i was analyzing the code more and trying to figure out why we did that but then i saw the method will only return a String. so if if we input a number or a object or some other weird stuff it would catch the error and using printstacktrace() it would pinpoint where the error is. and we use ioexception because this would be an instance of an input error. can someone comment whether i got it down right?
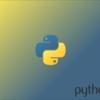
Kevin Faust
15,353 Pointshey rob,
my understanding is that we can only type strings in our inputs. in the below code, we have several string inputs(i put a few comments below). what if we typed a 3 or what if we typed in an object? isnt that where the "throw IOexception" comes into play? if we dont type a string, it should run the following:
System.out.println("Problem with input");
ioe.printStackTrace();
if this is not why we're doing the "throws IOException" and the try-catch blocks, then what exactly will trigger that catch block?
private String promptAction() throws IOException {
System.out.printf("There are %d songs available. Your options are: %n",
mSongBook.getSongCount());
for (Map.Entry<String, String> option :mMenu.entrySet()) {
System.out.printf("%s - %s %n",
option.getKey(),
option.getValue());
}
System.out.print("What do you want to do: ");
String choice = mReader.readLine(); //if we input anything other than a string, shouldnt it throw the exception?
// ^ right there it says String so only strings will be accepted
return choice.trim().toLowerCase();
}
public void run() {
String choice = "";
do {
try {
choice = promptAction();
switch(choice) {
case "add":
Song song = promptNewSong();
mSongBook.addSong(song);
System.out.printf("%s added! %n%n", song);
break;
case "quit":
System.out.println("Thanks for playing");
}
} catch(IOException ioe) { //if we dont type in a string in the inputs, shouldnt it run the following code?
System.out.println("Problem with input"); //itll print this
ioe.printStackTrace(); //itll show where the error was
}
} while(!choice.equals("quit"));
}
private Song promptNewSong() throws IOException {
System.out.print("Enter the artist's name: ");
String artist = mReader.readLine(); //if we input anything other than a string, shouldnt it throw the exception?
System.out.print("Enter the title: ");
String title = mReader.readLine(); //if we input anything other than a string, shouldnt it throw the exception?
System.out.print("Enter the video URL: ");
String videoUrl = mReader.readLine(); //if we input anything other than a string, shouldnt it throw the exception?
return new Song(artist, title, videoUrl);
}
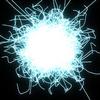
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Kevin, that makes more sense and I see what you're saying. We've basically told Java to expect a String which can be a lot in Java, when we input 3 from the requested input since Java was told to expect a string it is going to automatically assume that we mean "3" since it was told to expect a String. Really it's hard to not give Java string as input, even a single char of c will be read as "c" we put the catch method there because buffered reader is built to throw the exception so we had to have it for the method to compile.
The IOE exception really isn't there to catch a string so much as java does a very good job converting everything to a string, but let's say we change the method to only accept a char, or an int. Since there's no way for Java to change "My name is Greg" into an int, we would see the catch block ran, the same would be true a char, if it's expecting a single isntance of a letter if we typed "My name is Greg again" Java again has no way to convert this to a char.
But if we give it 3 since "3" is an appropriate String, java assumes that we mean that, so it accepts it as an input. Same with c since it owuld just be read as "c"
When you do the IOE exception it's more so to make that a String or char isn't passed in when you're looking for an int. Except we threw it in this instance because BufferedReader throws it, though to be honest if it's expecting a String it will have a very easy time converting things to the string. Which is again why it accepts 3 as the string "3"
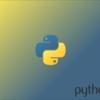
Kevin Faust
15,353 Pointshey rob,
ahhhhh that makes a lot of sense now and it cleared alot of my confusion. thanks alot rob!!! im going to add what you wrote to my notes
Kevin Faust
15,353 PointsKevin Faust
15,353 Pointshey rob thanks i understand
however im running the karaoke but even when i type in numbers im not getting an error. when it prompts for a "what do you want to do" and if i type in a number, itll just keept prompting until i type in "add" or "quit". and also when adding songs, i typed in the number 3 for the name and number 2 for the title and number 7 for the url and it says "Song: 2 by 3 added!"...i think something is wrong as it should be throwing errors.
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsRob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey Kevin u thought your question was more in general. The karokee isn't meant to throw the error because we don't set it up to. It really depends on the method. In the beginning it keeps looping until you enter one of the methods because its designed to do that. The trouble is there's too many things to pinpoint what code you could mean. I would recommend copying the code you have a question about. You should get more detailed answers that way.