Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial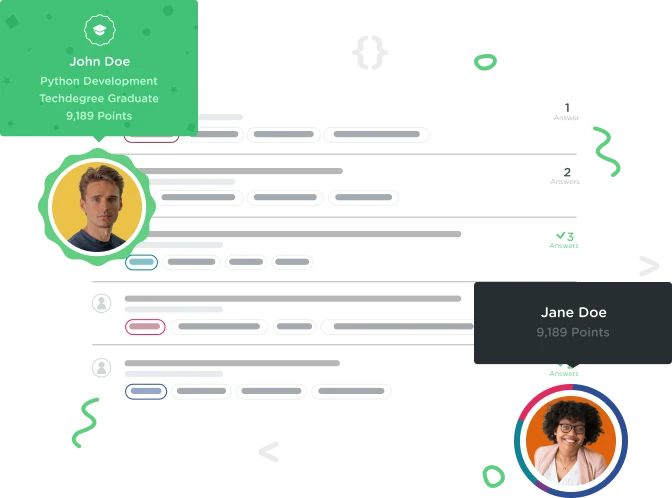
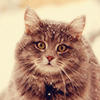
Jordan Amanda
2,275 PointsWhy/How does the list (guesses) "reset" after each run of game()?
So we create the variable list guesses and set it as an empty list. When we run game() we are adding the user's incorrect guesses to the list and we run a while loop until the list contains 5 or more incorrect guesses. This much I understand, but I'm stuck on why the list becomes empty again after each run of game(). Is this because changes to variables do not stay when they're changed in a loop?
def game():
# generate a random number between 1-10
secret_num = random.randint(1, 10)
guesses = []
while len(guesses) < 5:
try:
# get a number guess from player
guess = int(input("Guess a number between 1 and 10: "))
except ValueError:
print("{} isn't a number!".format(guess))
else:
# compare guess to secret number
if guess == secret_num:
print("you got it! My number was {}".format(secret_num))
break
elif guess < secret_num:
print("My number is higher than {}".format(guess))
else:
print("My number is lower than {}".format(guess))
guesses.append(guess)
else:
print("You didn't get it! My number was {}".format(secret_num))
play_again = input("Do you want to play again? Y/n ")
if play_again.lower() != 'n':
game()
else: print("Bye!")
game()
With my limited knowledge, I expected guesses to contain the guesses from previous runs of game() since we never explicitly "emptied" it, but this is not the case. Any help is appreciated.
1 Answer

osd
3,246 Pointsguesses will keep your previous guesses but in a different function stack. Essentially you calling the function game() inside of itself just starts a new stack of this function, or in other words starting it over. Which will then start the list guesses as an empty list. This is a new variable guesses that is unrelated to the previous.
Just to note, that since the function game() calls itself inside of the function, the stack will keep building on top of itself until you quit the game. Looking like this:
[initial game() call] [play again game() call][play again game() call]... until the you quit
So in each of these stacks there is a variable named guesses that has its own memory location and will hold your user input guesses for each game() call, but each will only hold your guesses from each individual game.
Hope this helps.
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsChanged comment to Answer.