Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial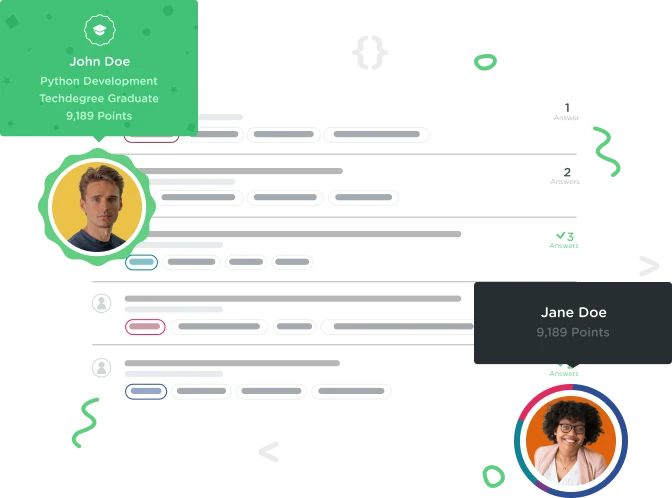
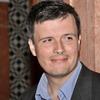
Brian Patterson
19,588 PointsWill not go to the profile page.
When I click on to login it will not go to the profile page. Here is my code for the index.js file.
var express = require('express');
var router = express.Router();
var User = require('../models/user');
// GET /profile
router.get('/profile', function(req, res, next) {
if (!req.session.userId) {
var err = new Error('You are not authorized to view this page.');
err.status = 403;
return next(err);
}
User.findById(req.session.userId).exec(function(error, user) {
if (error) {
return next(error);
} else {
return res.render('profile', {
title: 'Profile',
name: user.name,
favorite: user.favoriteBook
});
}
});
});
//GET /login route
router.get('/login', function(req, res, next) {
return res.render('login', { title: 'Login In' });
});
//POST login
router.post('/login', function(req, res, next) {
if (req.body.email && req.body.password) {
} else {
var err = new Error('Email and password are required.');
err.status = 401;
return next(err);
}
});
// GET /register
router.get('/register', function(req, res, next) {
return res.render('register', { title: 'Sign Up' });
});
// POST /register
router.post('/register', function(req, res, next) {
if (
req.body.email &&
req.body.name &&
req.body.favoriteBook &&
req.body.password &&
req.body.confirmPassword
) {
// confirm that user typed same password twice
if (req.body.password !== req.body.confirmPassword) {
var err = new Error('Passwords do not match.');
err.status = 400;
return next(err);
}
// create object with form input
/*
You'll notice that the value is coming from the request object.
So it's the information that the user filled out in the form.
*/
var userData = {
email: req.body.email,
name: req.body.name,
favoriteBook: req.body.favoriteBook,
password: req.body.password
};
// use schema's `create` method to insert document into Mongo
User.create(userData, function(error, user) {
if (error) {
return next(error);
} else {
return res.redirect('/profile');
}
});
} else {
var err = new Error('All fields required.');
err.status = 400;
return next(err);
}
});
// GET /
router.get('/', function(req, res, next) {
return res.render('index', { title: 'Home' });
});
// GET /about
router.get('/about', function(req, res, next) {
return res.render('about', { title: 'About' });
});
// GET /contact
router.get('/contact', function(req, res, next) {
return res.render('contact', { title: 'Contact' });
});
module.exports = router;
And here is my code for the profile page.
extends layout
block content
.main.container.clearfix
.row
.col-md-8.col-md-offset-2
h1.display-4
img.avatar.img-circle.hidden-xs-down(src='/images/avatar.png', alt='avatar')
| #{name}
h2.favorite-book Favorite Book
| #{favorite}
1 Answer
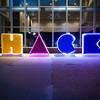
arshin
17,770 PointsWithin the profile GET route:
Use this: return res.render('Profile', { title: 'Profile', name: user.name, favorite: user.favoriteBook });
Rather than: return res.render('profile.....
.
First letter for profile should be capitalized.