Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial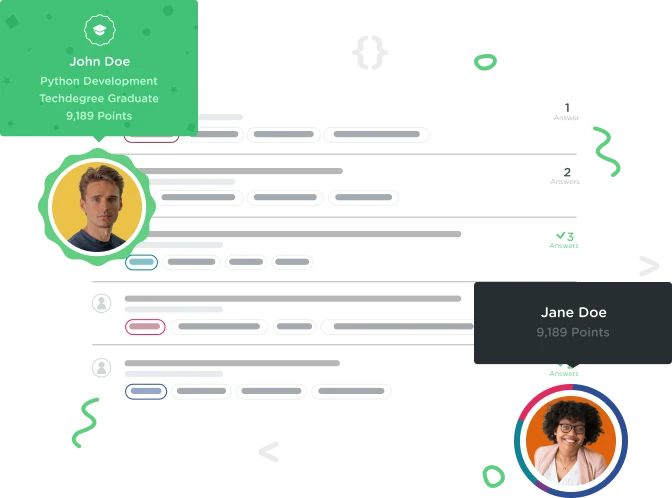

Hayden Bradfield
1,797 PointsWill someone explain to me what these "for" statements mean when traversing (JS to-do list course)?
Everything was fine and understandable until he got to the traversing lesson and now I am lost. I don't know what these "for" statements do with my code (towards the bottom of the code).
//Problem: User interaction doesnt provide desired results
//Solution: Add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task");
var addButton = document.getElementsByTagName("button")[0]; //First Button
var incompleteTasksHolder = document.getElementById("incomplete-tasks");
var completedTasksHolder = document.getElementById("completed-tasks");
var addTask = function() {
//Add a new task
console.log("Add Task...");
//When the button is pressed
//Create a new list item from #new-task
//input checkbox
//label
//input (text)
//button .edit
//button .delete
// Each elements needs modified and appended
}
var editTask = function() {
//Edit an existing task
console.log("Edit Task...");
//When the edit button is pressed
//if li is .editmode
//Switch from .editmode
//label text become input value
//else
//Switch to .editmode
//input value becomes label's text
//Toggle .editmode on the parent
}
var deleteTask = function() {
//Delete an existing task
console.log("Delete Task");
//When delete button is pressed
//remove parent list item from the unordered list
}
var taskCompleted = function() {
//Mark a task as complete
console.log("Complete Task...");
//When the checkbox is checked
//Append the task list item to the #completed-tasks
}
var taskIncompleted = function() {
//Mark a task as incomplete
console.log("IncompleteTask...");
//When checkbox is unchecked
//Append the list item to #incomplete-tasks
}
// WIRING
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("Bind list items...");
//select its children
//bind editTask to the edit button
//bind deleteTask to delete button
//bind checkBoxEventHandler to checkbox
}
//Set the click handler to the addTask function
addButton.onclick = addTask;
//cycle over incompleteTasksHolder ul list items
for (var i = 0; i < incompleteTasksHolder.children.length; i++) {
//for each list item
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[1], taskCompleted);
}
//cycle over completedTasksHolder ul list items
for (var i = 0; i < completedTasksHolder.children.length; i++) {
//for each list item
//bind events to list item's children (taskIncompleted)
bindTaskEvents(CompleteTasksHolder.children[1], taskIncompleted);
}
1 Answer
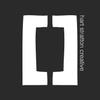
Angelica Hart Lindh
19,465 PointsHey Hayden,
You should think of a for
loop as something that is going to execute in your code over and over again until a condition is met in order to break out of the loop.
So in the case of your code, if we take for example the below loop:
//cycle over incompleteTasksHolder ul list items
for (var i = 0; i < incompleteTasksHolder.children.length; i++) {
//for each list item
//bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[1], taskCompleted);
}
When the javascript renderer reaches the point where for
is declared it will assess the arguments within the parentheses (var i = 0; i < incompleteTasksHolder.children.length; i++)
. This statement sets up a variable called 'i' and assigns the value zero to it. Next it checks if 'i' is less than incompleteTasksHolder.children.length and if the condition returns true, it will enter the code block within the { }
and execute the function within it.
In your case your loop is finding an <ul>
tag and iterating over each of the children and executing the bindTaskEvents()
function on each list item until it has reached the end of the list. E.g.
<ul>
// When the 'for' loop starts "var i = 0"
<li>Apple</li>// "i = 0"
<li>Orange</li>// "i = 1"
<li>Banana</li>// "i = 2"
<li>Pear</li>// "i = 3"
// "var i = 3" which is the length of the <ul>'s children <li> tags so the for loop breaks out and stops iterating
// !Remember index always starts at 0
</ul>
Hope this helps. If it is too trivial I apologise, but if it is please post some more code and the link to the course so I can get a deeper understanding of what is trying to be achieved and what the DOM tree looks like.
Hayden Bradfield
1,797 PointsHayden Bradfield
1,797 PointsThis is from the video in the full stack javascript track. It is the course "Interactive Webpages with Javascript"
Why did he choose the index of [1] and not all of them?
Angelica Hart Lindh
19,465 PointsAngelica Hart Lindh
19,465 PointsYou should use
.children[i]
as your reference not 1. Andrew states it in the course around minute 9.00 in the course.