Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial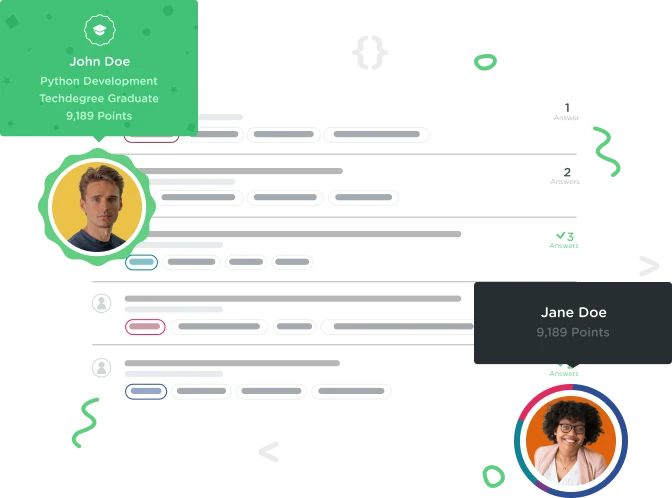

Ryan Van Den Bos
3,447 PointsWill the following php code send my html form data via email?
I have created a HTML form and want to process it and send it via email to my account however i have been having difficultly getting it to work, could you please look at my code and tell me wether i am on the right track or not?
I have changed my code following the treehouse Tutorial however it is not redirecting or sending can an body help ?
<?php
//makes sure the subbmision is set to post
if ($_SERVER["REQUEST_METHOD"] == "POST") {
//trim gets rid of any white space
$name = trim($_POST["Full_Name"]);
$email = trim($_POST["E-Mail"]);
$phone = trim($_POST["Phone_Number"]);
$message = trim($_POST["Query"]);
//makes sure form fields are not blank
if($name == "" OR $email == "" OR $phone == "" OR $message == ""){
echo "Please enter information for all the form fields.";
exit;
}
//prevents attack from hijackers
foreach($_POST as $value){
if(stripos($value,'Content-Type:') !== FALSE){
echo"There was a problem with the information you entered.";
exit;
}
}
//makes sure bots are not filling in form submission
if($_POST["adress"] != ""){
echo"Your form submission has an error";
exit;
}
//checks wether the email address is valid
if (!$mail->ValidateAddress($email)){
echo"Your email adress is not valid";
exit;
}
//email body
$email_body = "";
$email_body = $email_body . "NAME : " . $name . "\n";
$email_body = $email_body . "E-Mail : " . $email . "\n";
$email_body = $email_body . "Phone Number : " . $phone . "\n";
$email_body = $email_body . "Query : " . $message;
//imports third party php smtp email
require_once("class.phpmailer.php");
$mail = new PHPMailer();
$mail->IsSMTP();
$mail->SMTPAuth = true; // enable SMTP
$mail->Host = "smtp.live.com"; // sets the SMTP server
$mail->Port = 25; // set the SMTP port for
$mail->Username = "r1bos@hotmail.com"; // SMTP account username
$mail->Password = "****"; // SMTP account password
$mail->SetFrom($email, $name);
$mail->Subject = "Website Query";
$mail->MsgHTML($email_body);
$address = "r1bos@hotmail.com"; //to address
$mail->AddAddress($address);
if($mail->Send()) {
echo "Message sent!";
}
//redirects after submission to thank you page
header("Location: ../formconfirmation.html");
exit;
}
?>
?>```
```HTML
<form action="Php/form.php" method="post" name="Contact_Form" >
<label for="name">Full Name: </label>
<input type="text" name="Full_Name" id="name"><br>
<label for="email">E-Mail: </label>
<input type="text" name="E-Mail" id="email" ><br>
<label for="phonenumber">Phone Number: </label>
<input type="text" name="Phone_Number" id="phonenumber" ><br>
<label for="query">Query: </label>
<textarea name="Query" rows="4" cols="21" id="query" ></textarea><br>
<input type="submit" name="submit" value="Send" >
</form>```
1 Answer

Aaron Graham
18,033 PointsThe $_POST superglobal indexes your form elements based on name, not id. For example:
<input type="text" name="Full_Name" id="name">
could be assigned like this
<?php
$name = $_POST['Full_Name'];
?>
Also, I believe that php mail() uses sendmail or equivalent to actually handle sending the message. You probably want to make sure you have a working sendmail installation.