Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial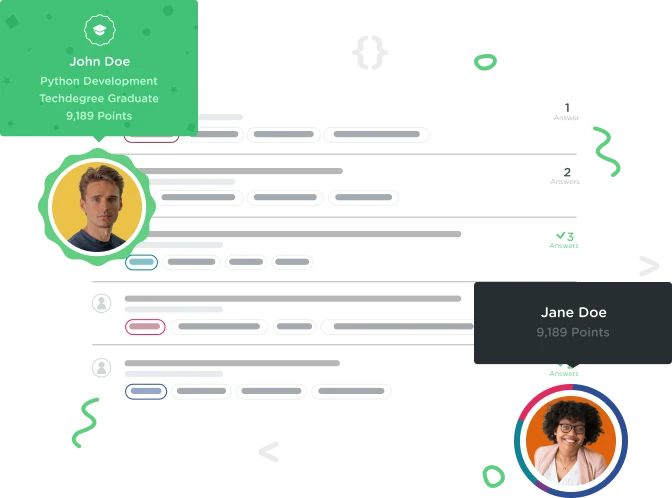
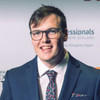
Simon Merrick
18,891 PointsWill the source map still work if I minify the css output?
For my project, I also want to minify the css after it has been compiled from sass. Will the source map still work after minification or would I have have to do the minification in the same task that I compile the sass, in between maps.init() and maps.write('./').
What about javascript?
3 Answers
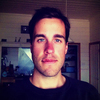
Sam Donald
36,305 PointsYou can do it from a single task
If you're going to serve minified files there isn't really any reason to generate individual css
files for each sass
file, generate maps
for each, and then compress to a single minified file that further extends it's map
to these individual css
files.
Why?
If you need to make changes you're still going to make them in your sass
files. Because any changes to these generated css
files would just be overridden/lost the next time you run these Gulp tasks (i.e. rebuild).
Instead
Just concatenate all your sass
into one minified css
file that gets exported to production. But still maps to each individual sass
file.
const gulp = require("gulp"),
sass = require("gulp-sass"),
rename = require("gulp-rename"),
minCss = require("gulp-clean-css"),
maps = require("gulp-sourcemaps");
gulp.task("compileSass", function(){
return gulp.src("_sass/main.scss")
.pipe(maps.init())
.pipe(sass())
.pipe(minCss())
.pipe(rename("everything.min.css"))
.pipe(maps.write("./"))
.pipe(gulp.dest("styles"));
});
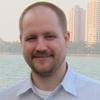
Ryan Field
Courses Plus Student 21,242 PointsHi, Simon. I believe it will, but because minification removes space/line breaks, I think you'll only see filename.scss:1
because technically it will all be on one line.
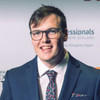
Simon Merrick
18,891 PointsI have done a bit more work on my gulp build pipeline and managed to resolve my problem, at least when dealing with my scripts. I found that passing {loadMaps: true} into sourcemaps.init() during the minify task seems to make sourcemaps use the first sourcemap as a reference to relate the minified file back to the very orignal source file. Bellow is an extract of my scripts section of my gulp build process
gulp.task("concatScripts", ['lintScripts'], function() {
return gulp.src([
config.paths.dep + '/jquery-1.11.3/jquery-1.11.3.js',
config.paths.dep + '/bootstrap/bootstrap.js',
config.paths.src + '/js/plugins.js',
config.paths.src + '/js/script.js'
])
.pipe($.sourcemaps.init())
.pipe($.concat('script.js'))
.pipe($.sourcemaps.write('./'))
.pipe(gulp.dest(config.paths.dist + '/js'));
});
gulp.task("minifyScripts", ["concatScripts"], function() {
return gulp.src(config.paths.dist + "/js/script.js")
.pipe($.sourcemaps.init({loadMaps: true}))
.pipe($.uglify())
.pipe($.rename('script.min.js'))
.pipe($.sourcemaps.write('./'))
.pipe(gulp.dest(config.paths.dist + '/js'));
});

Dan McCarty
10,082 PointsI can't say this is perfect, but...
When compiling scss partials to css, if you init your maps in that process and then load the maps (as in the previous example) during the minification process, the final maps will show where your source scss rules are (partial name and line).
Here's my working version:
gulp.task('sass-compile',function(){
return gulp.src('./scss/style.scss')
.pipe(maps.init()) // create maps from scss partials
.pipe(sass())
.pipe(maps.write('./')) // this path is relative to the output directory
.pipe(gulp.dest('./css')); // this is the output directory
});
// a task in here called concat-css concats all css files and changes name to output.css
gulp.task('minify-css',['concat-css'], function(){
return gulp.src('css/output.css')
.pipe(maps.init({loadMaps:true})) // create maps from scss *sourcemaps* not the css
.pipe(cleanCSS())
.pipe(rename('output.min.css'))
.pipe(maps.write('./'))
.pipe(gulp.dest('css'));
});