Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial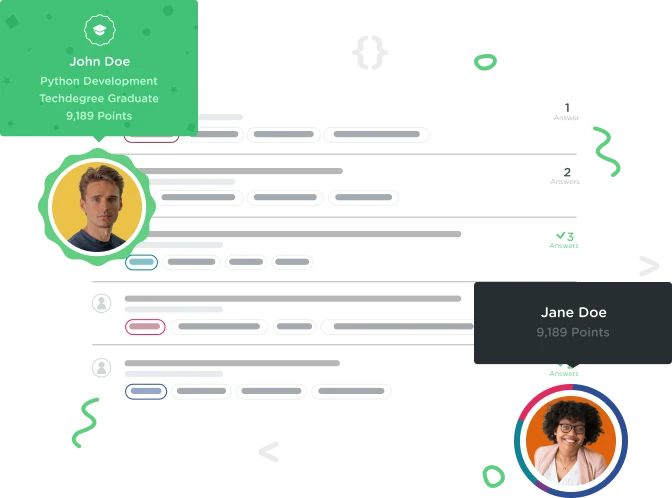

peter keves
6,854 Pointswith
what exactly is keyword "with" for ?
3 Answers
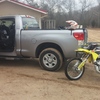
Ryan Ruscett
23,309 PointsHey,
Great questions. "with" will execute something within a context. mm Ok so what does that mean lol.
The "with" statement makes try/finally statements a whole lot easier.
Syntax is like this
with EXPRESSION as NAME:
BLOCK
The Expressions returns a value that the "with" statement uses to create a context. The context is what the block of code runs in. You need to import some stuff first.
The protocol that the "with" statement uses is called context management protocol. The object that implements this is the context managers.
Done like below.
from contextlib import contextmanager
@contextmanager
The @contextmanager is actually a decoration. Just know you use it before using the "with" statement. Lets not worry about what it does.
Ok, so still confused? heck, I am confused lol. Let's look at it like this.
I create a function that takes a file and a mode. Inside the function I use the open() function to open the file and return the object to f. This f variable or reference to an object is used to create the context. Then I do a try statement. Try some code. No matter what, if that code passes or fails or whatever. I want to use a finally at the end because even if it fails. I want to close the file. Or else I will never be able to open it back up again later.
Like this example I actually have to remember to close f. Or else I will be in trouble.
def opened(filename, mode = 'r'):
f = open(filename, mode):
try:
Do some code
finally:
f.close()
The "with" statement I don't have to worry. I don't even need try or finally anymore. Take this example
from contextlib import contextmanager
@contextmanager
with opened("/opt/toast") as f: <----- Opens file toast and puts it in the object into f used to create the context
for line in f: <----- iterates through the file f and prints the line stripped.
print line.strip()
Now what happens if print line.strip() fails. What if EOF error or whatever occurs. I didn't close the file did I? Well, I don't have to when I use the "with" statement. "with" creates a context. Now when I run my block of code on f. No matter what happens, the context will have the code to clean up any messes that occur. I don't have to remember to do it manually in the code. It just happens with python magic.
Still confused? One last example!
Think of the "with" statement.
We know that to drink milk. We must open it, drink it, close it and if not finished put it in the fridge or if finished put it in the trash.
What if the with statement said, open that jug of milk. Then the jug of milk becomes the context. The context already knows all about milk. So it knows that the top must go on, it must go in the fridge or if it's empty it has to go in the trash. So no matter what happens to you during the attempt to drink a jug of milk. Whether you pass, finish it and throw it in the air out of celebration. The context will handle closing it and putting it away if you pass out during the process and or catching it in mid air and throwing it away for you. You don't have to tell the context to do this. It just knowssss.
A computer only knows what we tell it. So typically I would have to explicitly say what I wanted to happen. Not anymore. Not with with and a context. It will all just happen for me.
It's just like using try with resources in Java if you are more familiar with that.
Does this make sense at all? It's typically an advanced topic and I am not sure of your skill level. So it's hard to talk about other better examples like generators or iterator objects returning. Since that may confuse you more. Do you understand the concept thought? If not please let me know if I can clear anything up .
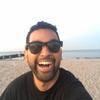
Anthony Albertorio
22,624 PointsFor some Jupyter notebooks to help understand Python see: https://github.com/tesla809/intro-to-python-jupyter-notebooks
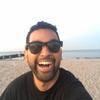
Anthony Albertorio
22,624 PointsHere is a great explanation of the with
statement and its associated magic methods aka dunder methods.
http://effbot.org/zone/python-with-statement.htm
peter keves
6,854 Pointspeter keves
6,854 Pointsthanks :)) !
Cem Ozer
3,013 PointsCem Ozer
3,013 PointsAre there any courses or tracks on Teamtreehouse that covers these advanced topics on Python such as generators, iterator objects or with?
SC3 Rocks
33,474 PointsSC3 Rocks
33,474 PointsCem Ozer, Ryan Ruscett
I would also like to know.